Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial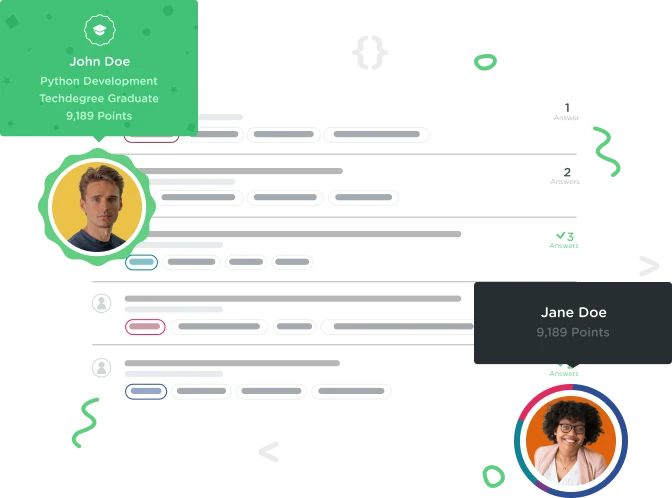

Charles Rotenberg
736 PointsCan't figure it out.
I've tried many ways of throwing the exception but never got it right. If someone could point out where and how to do or just give me a hint that would be very much appreciated.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer
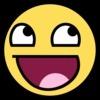
Michael Hess
24,512 PointsHi Charles,
One way that we can do this is to use an if-statement to check if the number of laps exceeds the number of battery bars. If the number of laps exceeds the number of battery bars we throw a new illegalArgumentException. If the number of laps is less than the bars count the number of laps is subtracted from the bars count ( mBarsCount -= laps).
public void drive(int laps) {
if (laps > mBarsCount){
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount -= laps;
}
If you have any other questions feel free to ask! Hope this helps!
Charles Rotenberg
736 PointsCharles Rotenberg
736 PointsIt worked! Thanks for the help!
Michael Hess
24,512 PointsMichael Hess
24,512 PointsMy pleasure -- glad I could help!