Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial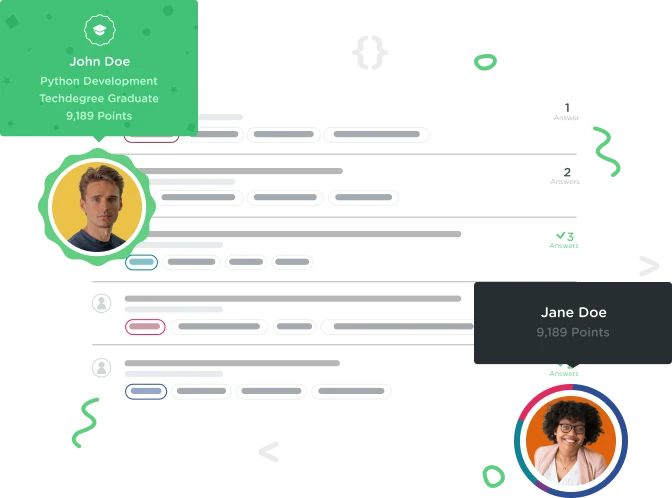

kevinthecoder
10,791 PointsCan't figure out first Javascript Code Challenge with Secret.
Ouch, I just started this new section and I'm stumped already! I watched the video three times so I must be missing something. Can anyone help please? What am I doing wrong?
var secret = prompt("What is the secret password?");
while (secret !== "sesame") {
alert(secret);
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
2 Answers
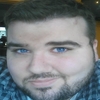
Marcus Parsons
15,719 PointsHey Kevin,
What you have to do is break out of that while loop. The condition for breaking out of the while loop is to have the variable secret
eventually be "sesame". Since the user is the one guessing what the password is, you have to keep prompting for a password each time the answer is not "sesame". If you prompt inside the while statement, the secret
will become "sesame" when the user guesses "sesame". In other words, all you have to do is prompt for the password and set it to be stored in the secret
variable in the while statement so that it can eventually break out once the correct password is guessed like so:
var secret = prompt("What is the secret password?");
while (secret !== "sesame") {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");

Jason Anello
Courses Plus Student 94,610 PointsHi Kevin,
You have an infinite loop if the user gets the password wrong the first time. It will just alert the secret over and over again. Your while loop needs a way to change the value of secret
so that eventually the condition will be true and you can break out of the loop.
The instructions ask you to continue prompting for the secret password until they get it right. So rather than alerting the secret to the screen you want to prompt for the password again and store the value entered in secret
.
kevinthecoder
10,791 Pointskevinthecoder
10,791 PointsInteresting. Yesterday, I just finished the (older) Ruby Foundations course as I had already done the Learn Ruby track a few weeks ago and was looking to get even more Ruby practice in. Maybe I was getting confused between Ruby and Javascript as there are so many methods, classes, loops etc.
These while loops are confusing and I can tell I'm already going to have a tough time with this. Marcus, I do see your completed code above and I will test it shortly. Here is my problem - when I see that code, all I 'see' in my brain is that we are SETTING secret to be a value (in this case, it's prompting user for input). On top of that, at least to me anyway, is that we ALREADY setting secret to the same value outside and before the loop! (look at the first line of code where we create the variable secret).
My brain instead WANTS to do an If statement. Example: "If" the prompted data the user enters is equal to "sesame", then the condition is true etc.
Hmm....confusing indeed. Sometimes, I get narrow tunnel vision and can only 'think' a particular way/direction. Not sure how I'm going to resolve this. Heck, I don't even know if what I wrote above will even make sense to you (and MOD Jason).
For the record, I'm trying to make a career transition into Web Development (I'm not sure what angle or niche yet, hence the need to learn as much Dev material as possible and see what sticks and/or see what I like/enjoy).
My other problem is that in my previous technical field, we needed to know EVERYTHING there is to know about a particular item/subject (sometimes referred to as a subject matter expert or SME for short) and we learn 100% of a particular area/item before we can 'interpret' that knowledge and then execute/do the mini-project for the customer/client etc. (look up Bloom's Taxonomy and look at the bottom two levels). I am stating this because I notice that with Web Development, there is SO MUCH material in SO MANY areas that it appears (to me at least) to be overwhelming AND that one can NEVER know EVERYTHING about just one particular small area in Web Development. I find this to be frustrating at times and am not sure at this early transition stage how best to proceed, learn new skills etc. I am trying my best to absorb material as quickly as possible.
How I get around all of this mess, I'm not sure yet. Suggestions are welcome, of course; then again, I'm probably rambling and perhaps my post needs to be in another area of the overall forum. I guess all I can do at this early stage is to take ONE section/problem at a time. Sorry for the long explanation..hahaha! :)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThe thing to remember is that if statements only execute once whereas a while (or do/while) loop can be executed as long as necessary. The first initialization of secret is the very first guess. If the very first guess would happen to be "sesame", the while loop would not even execute because it only executes while the loop condition is true. Once the loop condition becomes false, the loop will break and the rest of the code will continue. So, the loop condition is "while the secret variable is not equal to the string "sesame" continue executing the code within my code block". So, since this code is for guessing a password, we need to get new input each time and then check that it is not equal to "sesame" because of course once the secret variable becomes "sesame" the while loop becomes false and the code continues to the next line.
This is just an example of how while loops work and not something you'd put into production code.
If I can give you some advice for learning JavaScript, check out http://www.codeacademy.com and take their JavaScript course. It's totally free and highly interactive. It helped me a ton when I was first beginning.