Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial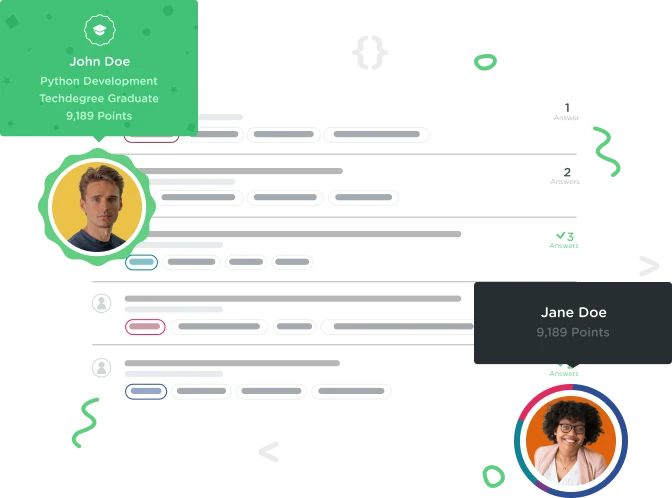
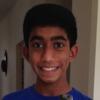
Sahil Ambardekar
10,306 PointsCant figure out how to complete code challenge
My code is attached, maybe 'open' is a reserved word?
class Store:
open = 9;
close = 10;
def hours():
return "We're open from {} to {}.".format(open, close);
1 Answer
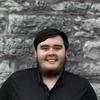
Michael Hulet
47,912 PointsYour problem is that you forgot to add the self
keyword where it needs to be. You need the hours
method to take it as a parameter, and then you need to prepend it to your references to open
and close
inside of the hours
method. This worked for me:
class Store:
open = 9
close = 10
# Notice the use of the self keyword in the below lines
def hours(self):
return "We're open from {} to {}.".format(self.open, self.close)
Also, on a side note, you should not use semicolons in Python, except when you're separating multiple commands on the same line, which you shouldn't really be doing. 99% of the time, you should give each statement its own line