Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial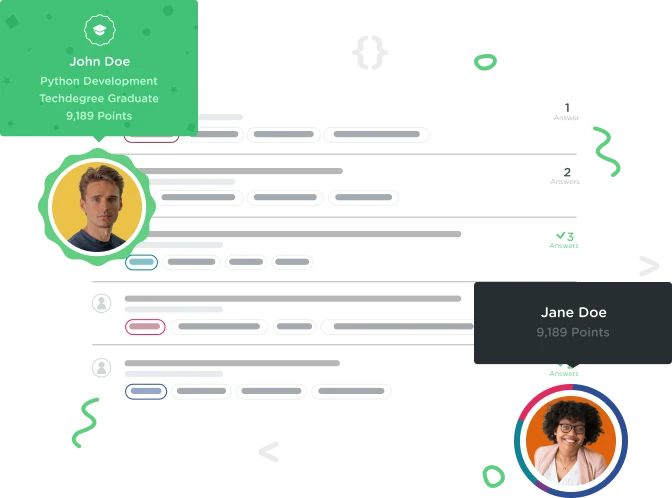

Jovy Ong
Front End Web Development Techdegree Student 14,858 PointsCan't figure out how to get the count of a category..
I'm currently stuck in this challenge where I need to return a Map<String, Integer> where the Integer is the count of the categories. I don't have any idea on how to get the count of each of the categories in the collection of BlogPosts.
My code so far:
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
public class Blog {
List<BlogPost> mPosts;
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> map = new HashMap<String, Integer>();
int count = 0;
for(BlogPost b: mPosts.toArray(new BlogPost[0])) {
map.put(b.getCategory(), 1);
}
return map;
}
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
1 Answer

Wikus van der Westhuizen
Courses Plus Student 4,335 PointsYou are on the right track. I'm a bit reluctant to just give you the answer since you are so close to it. So instead I'll point you in the right direction.
https://docs.oracle.com/javase/7/docs/api/java/util/Map.html
The link above is for the documentation for the Map class. Have a read through to see if it has anything that can help you.
SPOILER ALERT
Have a look at "containsKey(Object key)" and "get(Object key)"
Wikus van der Westhuizen
Courses Plus Student 4,335 PointsWikus van der Westhuizen
Courses Plus Student 4,335 PointsPS: Not sure they covered this in your course yet but you can update your for loop to use less code:
for(BlogPost b: mPosts)
Jovy Ong
Front End Web Development Techdegree Student 14,858 PointsJovy Ong
Front End Web Development Techdegree Student 14,858 PointsThanks for pointing me to the right direction. Just completed the challenge now using the get(); method.
Also thanks for pointing out that I don't have to convert the List<> to an Array to loop through it. This wasn't pointed out in the videos I've watched so far. Instructor always converted it to an Array in his demos.