Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial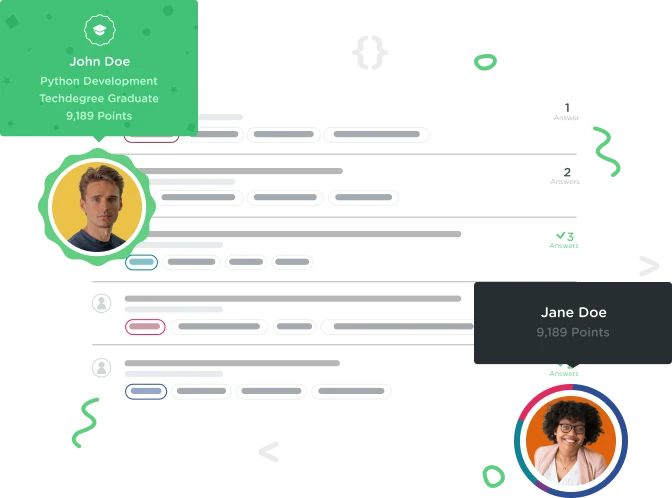

Dylan Chuckry
2,841 PointsCan't figure out how to remove items from a list by index.
So I am trying to complete this challenge.
I am hoping to create a list from the word then loop through each letter while comparing to the not allowed vowels. Next I want to remove the letters that don't follow the rule then .join the list back into a single script word. I am able to join the list back up and identify the error characters, however a problem arises when I try to remove items from the list. I keep getting a value error. Should I be raising an error and removing later instead of attempting to delete it right in the if statement?
I know I can find the solution if I tried but I do want to figure it out on my own. Any guidance would be greatly appreciated!
PS: I know my function doesn't have the right name, I am working on it in workshop to diagnose my issues easier then I pasted it over.
na_letter_low = ['a','e','i','o','u']
na_letter_up = ['A', 'E', 'I', 'O', 'U']
def d_vowel(word):
split_word = list(word) #duplicating word variable to not edit original
for letter in split_word: #creating loop to run through and test each letter in the word
index = 0 #Creating an index variable to scan through not allowed vowels
while index < 6: #looping through all vowels on first letter in word
if letter == na_letter_low[index] or na_letter_up[index]:
split_word.pop(index)
else:
index += 1
return "".join(split_word)
print(d_vowel("HelloWorld"))
2 Answers
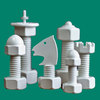
Steven Parker
231,624 PointsThis line caught my attention at first glance:
while index < 6:
You won't know what string the challenge will use to test your function, so you certainly can't assume it will have exactly 6 letters when converted.
Also, it's not a good idea to modify an iterable while it is controlling a loop, that can cause items to be skipped over.

Dylan Chuckry
2,841 PointsI meant to have .ammend. I ended up getting it, thank you
Dylan Chuckry
2,841 PointsDylan Chuckry
2,841 PointsThank you for your help, I made some edits but I can't figure out how to remove the item out of the loop.
Steven Parker
231,624 PointsSteven Parker
231,624 PointsI don't know what you were intending to do with "marked" but you create it as an empty list. And since you never add anything to it, there's nothing in it to remove.