Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial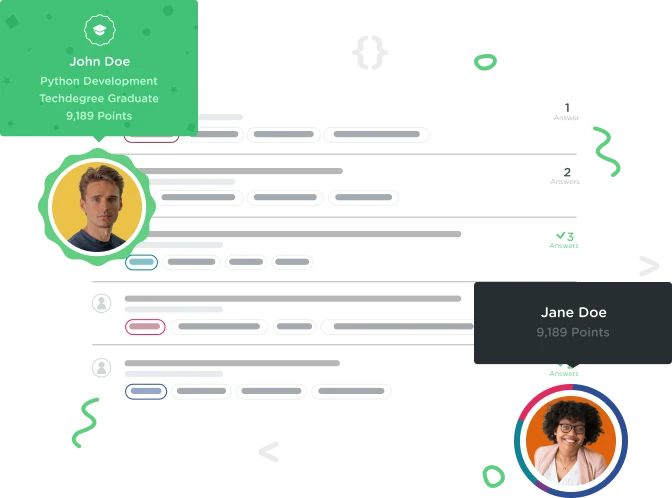
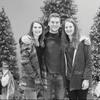
Garrett Hughes
Python Development Techdegree Student 32,971 PointsCan't figure out OOP instances challenge
I keep getting "TypeError: sequence item 0: expected str instance, FakeFloat found". How do I get around the fakefloat?
#Assuming x will be something like: ["apple", 5.2, "dog", 8]
#defining function
def combiner(x):
#variable for numbers
adder = 0
#list for the words
news = []
#Looping through x
for i in x:
#If 'i' is an int, float, or str continue, else do nothing with i.
if isinstance(i, (int, float, str)):
#if 'i' is a float or int add it to adder
if type(i) is int or type(i) is float:
#add numbers together
adder += i
#else append the str to the list
else:
news.append(i)
#add the number to the end of the list as a string
news.append(str(adder))
#Join everything together
joiner = "".join(news)
#return it
return joiner
2 Answers
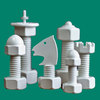
Steven Parker
230,274 PointsThis might not be a good place to use type().
When reading the documentation for type(), I found this recommendation: "The isinstance()
built-in function is recommended for testing the type of an object, because it takes subclasses into account." Combining that with the warning of the challenge itself "Be sure to use isinstance
to solve this as I might try to trick you." gives me the strong impression that in the actual test data there is at least one item subclassed (apparently as a "FakeFloat") in a way that would fool type().
You're very close, and if you refactor your code to make all the type determinations using isinstance() you should have no trouble passing the challenge.

Justin Wampler
4,523 PointsFor anyone wondering how to shorten the code, one can use list comprehensions. Something like this:
def combiner(list_):
return (''.join([item for item in list_ if isinstance(item, str)]) +
str(sum([item for item in list_ if isinstance(item, (int, float))])))