Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial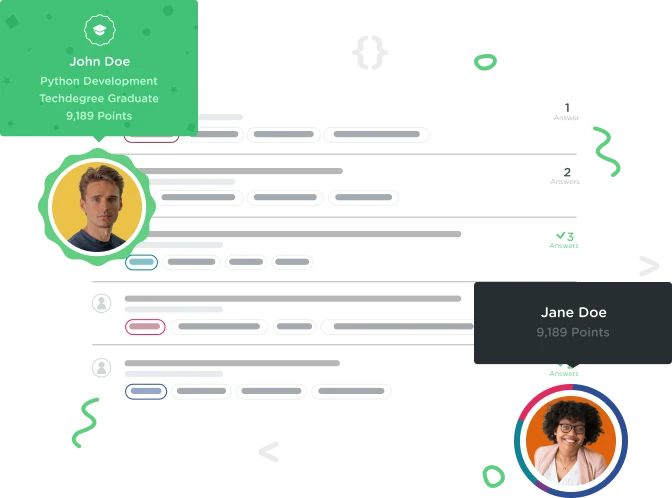
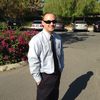
Richard Parkins IV
1,970 PointsCant Figure out what to do Keeps saying make sure your adding an instance Method called getFullName().
Not sure how to make this work or what i did wrong..
struct Person {
let firstName: String
let lastName: String
func getFullName(firstName: String, lastName: String) {
let fullPersonName: String = "\(firstName) " + "\(lastName)"
return
}
}
let newPerson = Person(firstName: "", lastName: "")
newPerson.getFullName("Richard ", lastName: "Parkins")
2 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsHey there! Let's walk through the code :)
struct Person {
let firstName: String
let lastName: String
// 1. You don't need the parameters here, as we are using
// the firstName and lastName of this very struct
// 2. The function is supposed to return a String, but you have
// not defined a return type, so it won't return anything.
// You specify the return type via -> Type after the parameter
// definition. See code below.
func getFullName(firstName: String, lastName: String) {
// It's not necessary to concatenate Strings here, though possible.
// As you are already using string interpolation, let's merge it to
// one String. See code below.
let fullPersonName: String = "\(firstName) " + "\(lastName)"
// Now you have to return fullPersonName, this is returning
// "nothing" and the compiler would not let you as there is
// no return type specified for this function, as mentioned before
return
}
}
// 1. Why create a Person object that has empty names.
// Specify the names right here
// 2. The assignment asks you to create a constant called aPerson,
// not newPerson. It is vital to stick to the assignment description,
// otherwise you won't pass the challenge even if your code works
let newPerson = Person(firstName: "", lastName: "")
// As you have not defined a return type before, you can't assign
// a return value, so this method does nothing, basically.
// Moreover, as mentioned before, there's no need for passing
// the names here, as you can use the ones you initialized the
// struct with
newPerson.getFullName("Richard ", lastName: "Parkins")
Taking into account what I said above, the code could look something like this
struct Person {
let firstName: String
let lastName: String
// No parameters, returns a String
func getFullName() -> String {
// You can also return the string right away
return "\(firstName) \(lastName)"
}
}
// Let's use the struct to create an instance of Person and assign it to a constant named aPerson
let aPerson = Person(firstName: "Richard", lastName: "Parkins")
// Once you have a Person instance, call the instance method and assign the full name to a constant named fullName.
let fullName = aPerson.getFullName() // Richard Parkins
Hope that helps :)
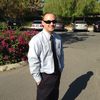
Richard Parkins IV
1,970 PointsThanks guys!! I didn't realize that a method already has access to the constants that are set in the Structure. A good thing to remember. Also the reason i was doing the string interpolation and concatenation was to add the space between the first name and the last name because it said (make sure you leave a space between the first and last name.) That was totally unnecessary. Thank you guys for the help, I really appreciate it. I messed around with that for like two hours and couldn't get it to work.
Thanks again!