Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial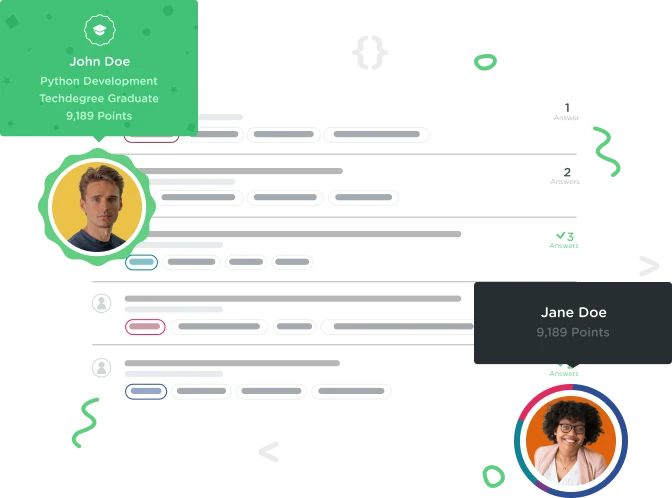

Anuj Sachdeva
13,034 PointsCan't figure out what's wrong with my code
Console shows no error but the code's still not working.
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i = 0; i < employees.length; i++) {
if (employees[i].inoffice === true){
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
The console doesn't even recognise the employee variable.
Here's the image of that weird error that's popping up the console
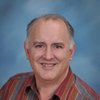
Mark Casavantes
Courses Plus Student 13,401 PointsHi Anuj,
This line has a missing semi-colon. statusHTML += '<li class="out">'
See if that works.
Mark

Anuj Sachdeva
13,034 PointsRokas Mazeika that part's there in the workspace but somehow got skipped in the question. I've updated my post now so I'd like you to take another look at it. I could really use another pair of eyes cos I can't find an error.

Anuj Sachdeva
13,034 PointsJP Chaufan Field, followed your advice and I'm even more confused as the console refuses to recognise xhr.readyState. Really can't figure out what's wrong with the code.

Anuj Sachdeva
13,034 PointsMark Casavantes, I added that semi-colon but it still didn't work :/
2 Answers

Rokas Mazeika
4,243 Pointsyour missing the document.getElementById('employeeList').innerHTML = statusHTML; in if statment

Rokas Mazeika
4,243 Pointsmaybe u didn't put <script src="js/widget.js"></script>
if not then try my code
var xhr = new XMLHttpRequest();
xhr.open("GET", "data/employees.json")
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
var employee = JSON.parse(xhr.responseText);
var statushtml = '<ul class="bulleted">'
for (var i = 0; i < employee.length; i++) {
if (employee[i].inoffice === true) {
statushtml += '<li class="in">'
} else {
statushtml += '<li class="out">'
}
statushtml += employee[i].name
statushtml += "</li>"
}
statushtml += "</ul>"
document.getElementById("employeeList").innerHTML = statushtml;
}
}
xhr.send();
JP Chaufan Field
1,655 PointsJP Chaufan Field
1,655 Pointsmaybe the readyState does not == 4? i might try printing out the readyState to see what numbers its being changed to