Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial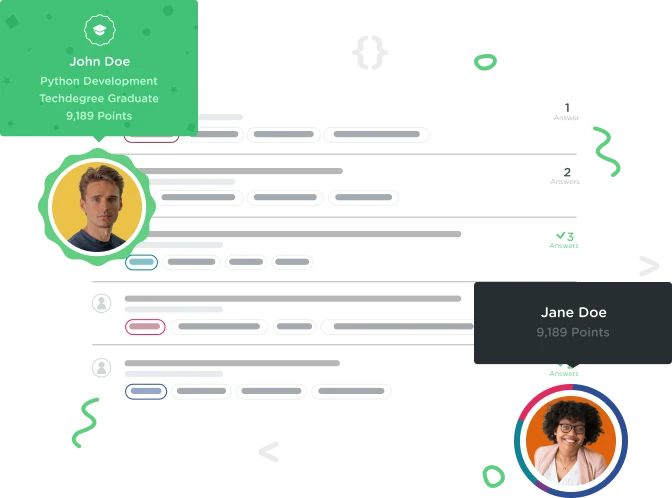

Alex Owen
10,642 PointsCan't figure out why my setter method isn't working
Hi there,
I'm really confused as to why this setter method isn't passing, does anybody have any suggestions?
Thanks
class Product:
_price = 0.0
tax_rate = 0.12
def __init__(self, base_price):
self._price = base_price
@property
def price(self):
return self._price + (self._price * self.tax_rate)
@price.setter
def price(self, price):
self._price = (price * 100) / (100 + (self.tax_rate * 100))
1 Answer

andren
28,558 PointsThe price setter is actually meant to be very simple, it should set self._price
to the passed in value and nothing else. All of the tax calculations happens in the getter so you don't have to do any calculations in the setter.
So the issue is that your setter does far more with the value than it should. If you simply set it like this:
@price.setter
def price(self, price):
self._price = price
Then your code will pass.
Alex Owen
10,642 PointsAlex Owen
10,642 PointsHi andren,
Thanks for your reply. This worked, but I still don't understand why. Wouldn't setting self._price to the value of the price argument result in the price being set into something different because of the calculations that occur under the @property decorator? I can't seem to understand why calculating the reverse percentage of the price value that you want and then setting that value to self._price is unnecessary. What am I missing?
Thanks
[MOD: escaped underscore to fix markup. -cf]
andren
28,558 Pointsandren
28,558 PointsThe calculations under the @property decorator only happens when you access the price from the object, not when you set it, and it producing a different price from the set price is intentional.
With the getter and setter methods in place you can set the price to whatever you want to sell a product for (without thinking about tax), and then when you get the price you get to see what the price is when tax is accounted for.
If you wanted to get the exact same price back that you set then using properties would be pointless, since you could just use a normal attribute instead which would be far simpler.