Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial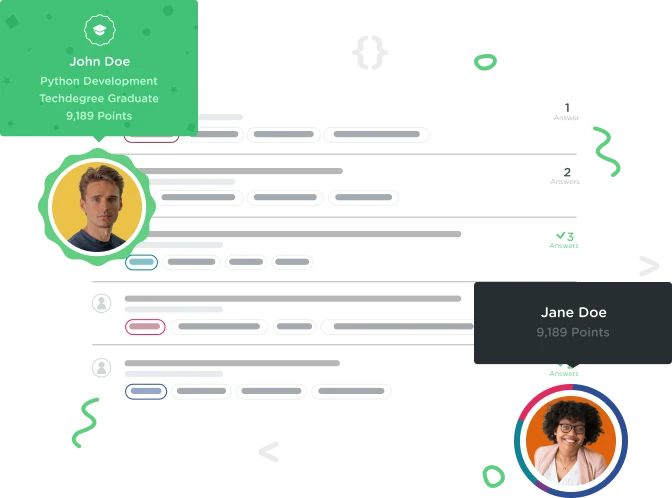

Jackson Monk
4,527 PointsCan't figure this one out
I am trying to make a program that takes user input of a name and turns their input into regular names, hyphenated names, nicknames, prefixes, and suffixes. All of it has gone well so far, except for nicknames. I tell the user to put their nicknames in quotes (single or double) and I will be able to print what they typed in the quotes. Example:
Input: Dwayne 'The Rock' Johnson Output: Regular Names: Dwayne, Johnson; Nicknames: 'The Rock'
I have made a loop to iterate through all the characters in the input and try and find nicknames, here it is:
// get nicknames
for (let a = 0; a < char.length; a ++) {
if (char[a] == "'" || char[a] == '"') {
joinarr.push(char[a]);
for (let b = a + 1; true; b ++) {
if (char[b] == char[a]) {
joinarr.push(char[b]);
break;
}
joinarr.push(char[b]);
}
}
}
Notice that the loop with 'a' as a counter loops through all the characters. In the loop, it tests If char[a] is a single or double quote. If it is, it adds that quote to the joinarr array. It then enters a loop with a true condition that uses 'b' as a counter, with a value of a + 1. It then tests if char[b] is equal to char[a]. If it is, that would mean that char[b] is the end quote for that nickname, so it adds the end quote to the array and breaks the loop, otherwise it just adds char[b] to the array. My problem is that when I run this, my program crashes, telling me that the true loop never stops which is strange because I always type the corresponding end quote. Been at this a couple days, anyone any ideas?
1 Answer
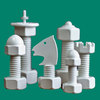
Steven Parker
230,274 PointsThe first time the "a" loop encounters a quote, the "b" loop will find a matching one. But the next time the "a" loop encounters a quote it will be the ending one and there will be no match.
You probably want to advance index "a" before you break out of the "b" loop ("a = b + 1
"). Then the outer scan will continue where the inner one left off.
But there's also no reason to allow the "b" loop to be unlimited and crash on a malformed input. A sensible limit would be to stop if the end of the string is reached ("b < char.length
").
Jackson Monk
4,527 PointsJackson Monk
4,527 PointsYou are right, it probably would be more sensible to make a limit rather than have it crash. Also thank you for the fix. I spent so much time focusing on b's index that I didn't think about a being stuck on the first quote. Thanks Steven