Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial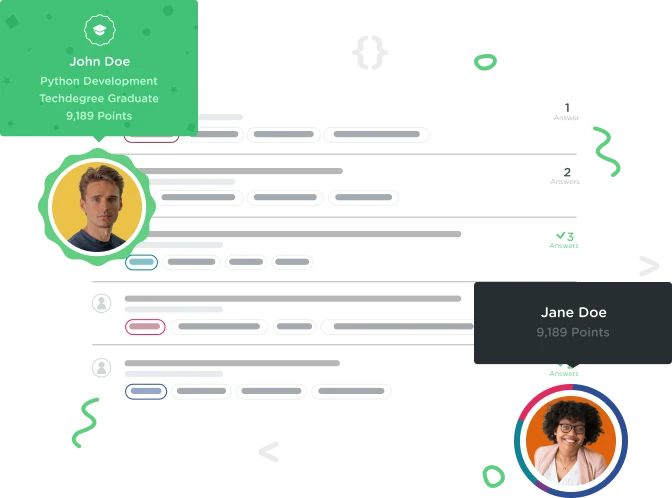

Theodor Parfene
3,125 PointsCan't figure this out at all
Can someone please tell me what I'm doing wrong here? My score doesn't go above 1, for some odd reason, even though I'm answering 2 or more correctly.
// This is a quiz program
var score = 0;
var answer1 = prompt('In which year was Einstein born?');
if (answer1 === 1879) {
score =+ 1;
}
var answer2 = prompt('A pineapple is a fruit or a vegetable?');
if (answer2.toUpperCase() === 'FRUIT') {
score =+ 1;
}
var answer3 = prompt('Alexander the Great was the prime minister of Japan. True/False');
if (answer3.toUpperCase() === 'FALSE') {
score =+ 1;
}
var answer4 = prompt('Bears can live up to 30 years. True/False');
if (answer4.toUpperCase() === 'TRUE') {
score =+ 1;
}
var answer5 = prompt('How many characters does this question have?');
if (answer5 === 'How many characters does this question have?'.length) {
score =+ 1;
}
document.write('Your score is ' + score);

Theodor Parfene
3,125 PointsHi, thank you! That was embarrassing, haha.
But I am now having trouble with the number type answers. (questions 1 and 5). Can you tell me what is wrong with those?

damiencouteillou
4,715 Pointsbecause of the ===, javascript is expecting a number and your passing a string in the console. So you only need to put 2 equals.

Theodor Parfene
3,125 PointsThanks again! That solved it. I need to review the videos..
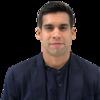
Johnny Garces
Courses Plus Student 8,551 Pointsalso for the number values (i.e. 1879) make sure you parseInt(answer1) as the number is a string at first. nice quiz!
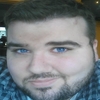
Marcus Parsons
15,719 PointsHey Johnny,
Read my comments about that in my answer below. It is much slower to use parseInt on a variable than it is to just compare it to a string.
2 Answers
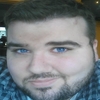
Marcus Parsons
15,719 PointsAs Damien mentioned, you can use == to do a less strict comparison operator. The == sign automatically converts variables from one type to another as in this case converting a string into a number and vice versa.
But, you should AVOID using ==. Instead, you should do the type conversion yourself, and use a strict operator for comparisons like so:
var answer1 = prompt('In which year was Einstein born?');
//use parseInt for integers
if (parseInt(answer1) === 1879) {
score += 1;
}
//Etc.
The following is a quote from Douglas Crockford's book JavaScript: The Good Parts.
"JavaScript has two sets of equality operators: === and !==, and their evil twins == and !=. The good ones work the way you would expect. If the two operands are of the same type and have the same value, then === produces true and !== produces false. The evil twins do the right thing when the operands are of the same type, but if they are of different types, they attempt to coerce the values. the rules by which they do that are complicated and unmemorable. These are some of the interesting cases:
'' == '0' // false
0 == '' // true
0 == '0' // true
false == 'false' // false
false == '0' // true
false == undefined // false
false == null // false
null == undefined // true
' \t\r\n ' == 0 // true
The lack of transitivity is alarming. My advice is to never use the evil twins. Instead, always use === and !==. All of the comparisons just shown produce false with the === operator."

Theodor Parfene
3,125 PointsHello,
That was some incredibly good advice and I thank you. I have forgotten about the parseInt() function. It is a bit confusing as to when to or if I should ever use the "evil twins", if I can use inbuilt functions to specify exactly what I want.
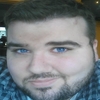
Marcus Parsons
15,719 PointsYou are very welcome, Theodor. The == sign is something you should avoid like the plague. There is no case where you can achieve something with a == that you can't with a ===. The == is just there for lazy programmers who don't want to bother with type conversion. And as you can see above in the logic test cases, it can result in some really hard to debug programs, especially when you get involved with lots of lines of code. Being strict with the program will make debugging a thousand times easier, and it will ensure that any future team you partner with will be sure to thank you, instead of curse you, if you get started with a good habit like being strict (on the program) now. :)
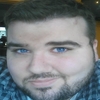
Marcus Parsons
15,719 PointsBy the way, I thought about it for another minute, and there's really no need to parse the integer from the answer variable because you aren't doing any mathematical manipulation with that number. Instead, just treat it a string and it will be much faster. I even ran a js performance test that you can see here and the results are that the string check is MUCH faster than parsing the integer. Moral of this story is don't parse unless you're going to manipulate!
var answer1 = prompt('In which year was Einstein born?');
if (answer1 === "1879") {
score += 1;
}
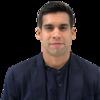
Johnny Garces
Courses Plus Student 8,551 Pointsgreat advice, Marcus. Thanks for sharing!
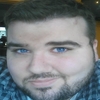
Marcus Parsons
15,719 PointsAnytime, Johnny!
damiencouteillou
4,715 Pointsdamiencouteillou
4,715 PointsHello,
You need to do score += 1 instead of =+ 1 I think