Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial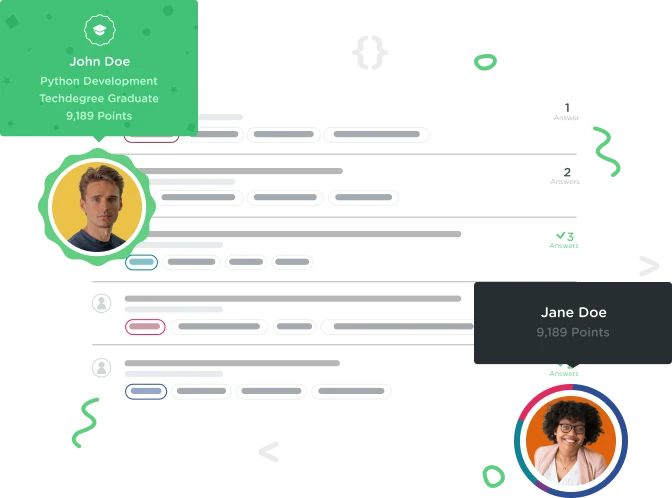
Miguel Angel Rodriguez
11,280 PointsCant fin the error! Please help
private static boolean copyFile(InputStream in, FileOutputStream out) throws IOException { // Copy magic intentionally omitted try { byte[] buffer = new byte[1024]; int read; while((read = in.read(buffer)) != -1){ out.write(buffer, 0, read); } } catch(FileNotFoundException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } return true; } }
import java.io.InputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) throws IOException {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
copyResult = copyFile(in, out);
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static boolean copyFile(InputStream in, FileOutputStream out) throws IOException {
// Copy magic intentionally omitted
try {
byte[] buffer = new byte[1024];
int read;
while((read = in.read(buffer)) != -1){
out.write(buffer, 0, read);
}
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
return true;
}
}
2 Answers

James Simshaw
28,738 PointsHello,
What you are currently trying to do is write the "copy magic" that "omitted" which you don't need to do for this challenge. It was just omitted from the screen, but is still present and called in the final product so it doesn't need to actually be written. Instead, you need to check if the output file exists in saveAssetImage and if it does, you want to do nothing, otherwise, you want to execute the try block in saveAssetImage.
Miguel Angel Rodriguez
11,280 PointsHi, I tried with the method "exists" before the Try
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
if (fileToWrite.exists()) {
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
copyResult = copyFile(in, out);
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The error said: "The file is not being copied. Identify the code that tries and add an "if" check around it. I tried with the if inside the "try" and nothing happens.
Thanks for help

James Simshaw
28,738 PointsYou're currently copying only if the file exists, but you want to only write it if the file does not exist.
Miguel Angel Rodriguez
11,280 Pointsjajaja thanks a lot :)
Sanat Tripathi
8,109 PointsSanat Tripathi
8,109 PointsCan you also comment the error that you are seeing?