Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial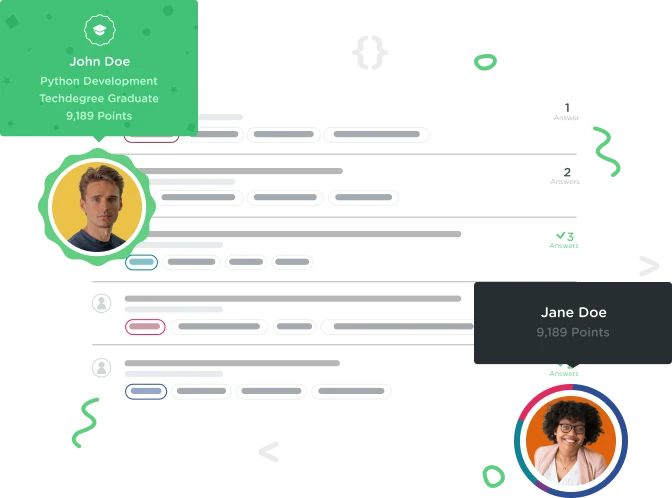

Samuel Cordova
807 PointsCan't find the correct answer to the last question on java basics.
Can't seem to answer the question that follows "Finally, using console.prinf print out a formatted string that says "Because you said<response>, you passed the test!"
My Code is below:
// I have initialized a java.io.Console for you. It is in a variable named console. String response; boolean isInvalidWord; do { response= console.readLine("Do you understand do while loops?" ); isInvalidWord = (response.equalsIgnoreCase("No")); if (isInvalidWord); { console.printf("Try again. \n\n"); } } while (isInvalidWord); console.printf("Because you said %s, you passed the test!", response); }
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
boolean isInvalidWord;
do {
response= console.readLine("Do you understand do while loops?" );
isInvalidWord = (response.equalsIgnoreCase("No"));
if (isInvalidWord); {
console.printf("Try again. \n\n"); }
} while (isInvalidWord);
console.printf("Because you said %s, you passed the test!", response);
}
3 Answers
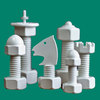
Steven Parker
241,783 PointsThere is stray extra closing brace on the very last line causing a syntax error. Just remove it and you'll pass.
And while your method works just fine, you don't really need to create an extra variable for testing, you can just include the test expression in the while directly, like this:
do {
response = console.readLine("Do you understand do while loops?");
} while (response == "No");

Shem Ogweno
15,393 PointsYou got it! remove the extra closing brace on the last line. Here is another way to solve it!
String response;
boolean isInvalidWord;
do {
response = console.readLine("Do you understand do while loops?");
isInvalidWord = (response.equalsIgnoreCase("No"));
} while (isInvalidWord);
console.printf("Because you said %s, you passed the test!", response);

Samuel Cordova
807 PointsThank you! I appreciate your help. The closing brace concept has been confusing me.

Samuel Cordova
807 PointsSteven Parker, wow! I see it now. Thank you so much for breaking it down like that! You're the best!
Samuel Cordova
807 PointsSamuel Cordova
807 PointsThank you! The way you have it is much easier. Do you know why the extra closing brace is not needed? I thought once we open up a code block we had to close it. That's been confusing for me.
Steven Parker
241,783 PointsSteven Parker
241,783 PointsYou're right, braces must always be used in matching pairs. So here's your code with comments added: