Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial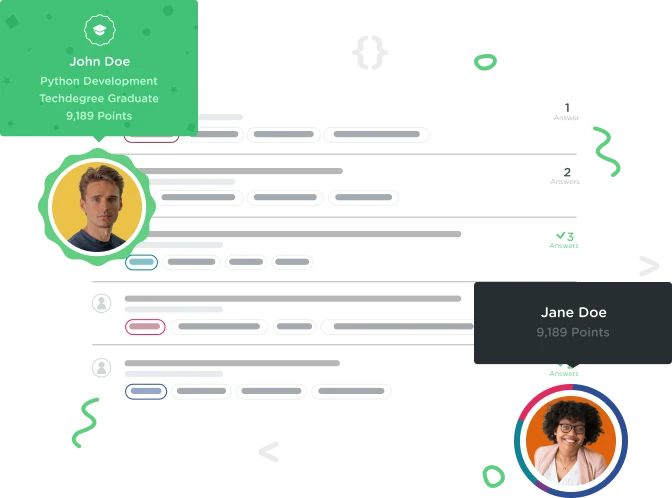

Tarek Singh
Courses Plus Student 3,653 Pointscan't find the solution
Can anyone help me out please
import datetime
dates = [
datetime.datetime(2012, 12, 15),
datetime.datetime(1987, 8, 20),
datetime.datetime(1965, 2, 28),
datetime.datetime(2015, 4, 29),
datetime.datetime(2012, 6, 30),
]
def is_2012(dates):
return dates.year == 2012
dt_2012 = list(filter(is_2012))
return dt_2012
1 Answer
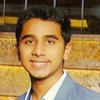
Anish Walawalkar
8,534 PointsHey Tarek, you were on the right track. Just a few pointers:
- When you use the filter(func, iterable) function you have to pass it a function and an iterable (in your case the iterable is a list of datetime objects)
- The challenge asks you to return a generator so you don't need to convert the result returned by the filter function into a list
- You should only return inside of a function
so in code the solution looks like this:
import datetime
dates = [
datetime.datetime(2012, 12, 15),
datetime.datetime(1987, 8, 20),
datetime.datetime(1965, 2, 28),
datetime.datetime(2015, 4, 29),
datetime.datetime(2012, 6, 30),
]
def is_2012(date):
return date.year == 2012
dt_2012 = filter(is_2012, dates)