Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial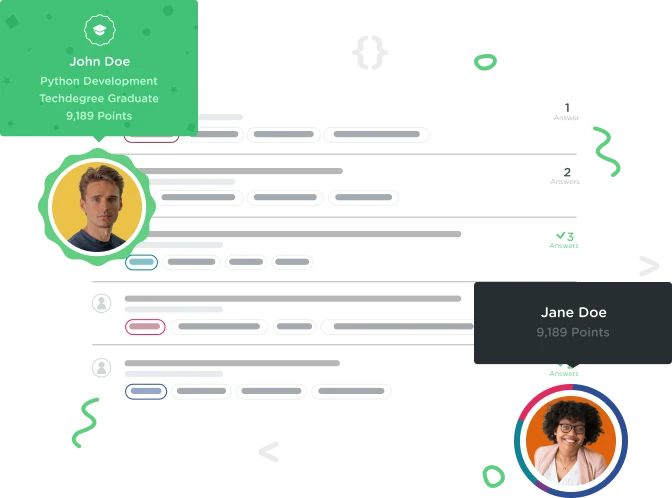
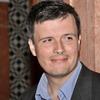
Brian Patterson
19,588 PointsCan't get css files to load or javaScript to work in Node.js server.
I am trying to get a simple Rock Paper Scissors app to work with a Node.js server. Here is my code for the server.js .
var http = require('http');
var fs = require('fs');
function onRequest(request, response) {
response.writeHead(200, {'Content-Type': 'text/html'});
fs.readFile('./index.html', null, function(error, data) {
if (error) {
response.writeHead(404);
response.write('File not found');
} else {
response.write(data);
}
response.end();
});
}
http.createServer(onRequest).listen(3000, function(){
console.log("The server has started");
});
Now it is rending the html file ; but is not rendering the CSS file or the JavaScript. What would I need to do to render the CSS file. Below is my html code.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Rock Paper Scissors Game</title>
<link rel="stylesheet" href="styles.css">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" media="screen" href="main.css" />
<script src="main.js"></script>
</head>
<body>
<header>
<h1>Rock Paper Scissors</h1>
</header>
<div class="score-board">
<div id="user-label" class="badge">user</div>
<div id="computer-label" class="badge">comp</div>
<span id="user-score">0</span>:<span id="computer-score">0</span>
</div>
<div class="result">
<p>Paper covers rock, You win!</p>
</div>
<div class="choices">
<div class="choice" id="p">
<img src="images/paper2.png" alt="paper">
</div>
<div class="choice" id="s">
<img src="images/scissors2.png" alt="scissors">
</div>
<div class="choice" id="r">
<img src="images/rock2.png" alt="rock">
</div>
</div>
<p id="action-message">Make your move</p>
<script src="app.js"></script>
</body>
</html>
Any help would be appreciated.
2 Answers

Neil McPartlin
14,662 PointsHi Brian. Files such as css and pngs etc. are regarded as 'static' or 'public' files. You probably found that when simply viewing your index.html on your local PC, (without Nodejs), these files were loaded automatically and it was your browser that handled that for you. But with nodejs, you have currently only coded for index.html, so that in your browser, you can see at http://localhost:3000/ your index.html only.
In your browser, if you look at your JavaScript console (F12 in Chrome), you will see these 2 errors (and maybe more)...
Resource interpreted as Stylesheet but transferred with MIME type text/html: "http://localhost:3000/styles.css".
Resource interpreted as Stylesheet but transferred with MIME type text/html: "http://localhost:3000/main.css".
Your browser recognises that these 2 files are required but the node server cannot currently handle the request. Instead the server just returns the index.html file which the browser knows is not formatted as text/css.
So you would need to manually add 'routes' for each of these static files. In a nutshell, it can be done in native nodejs but quite quickly you would find this is not the way to go. Instead, this is why frameworks like 'Express' are so popular because they have tools built-in, that handle all of this for you.
Here are just 2 links that show how complex this can get if you try and do this manually.
https://stackoverflow.com/questions/38295722/how-do-you-serve-css-in-a-node-js-application
https://teamtreehouse.com/community/cant-get-the-css-to-load-in-the-nodejs-server
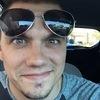
Caleb Frieze
29,582 PointsYou'll want to make sure you have the correct path to your CSS file, as well as making sure the CSS file is accessible relative to your index.html file.
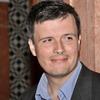
Brian Patterson
19,588 PointsCan you explain.