Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial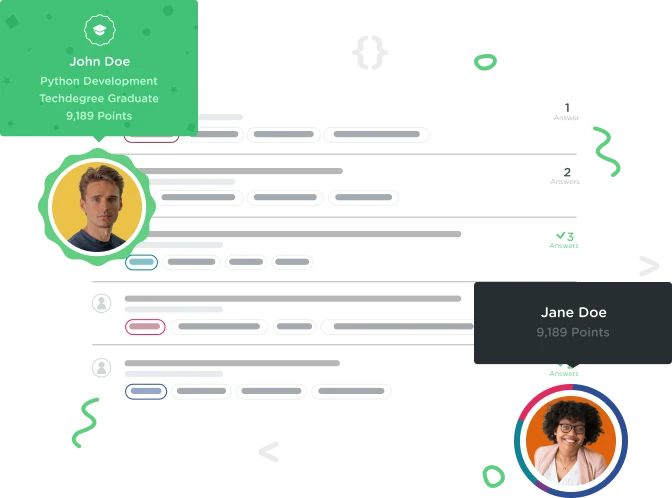

Kristian Mazsar
3,936 PointsCant get it right, i dont know what ID i should use :( help
Am I wrong at the Bind View or something ?? Please heelp
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import android.widget.ImageView;
public class MovieActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
@BindView(R.id.linearLayout) TextView mTitleLabel;
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white" >
<TextView
android:id="@+id/movieTitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="32sp"
android:text=""
android:layout_centerHorizontal="true"
android:layout_centerVertical="true" />
<ImageView
android:id="@+id/movieImage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@id/movieTitle" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/movieTitle"
android:layout_centerHorizontal="true"
android:id="@+id/linearLayout">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/textView"
android:layout_weight="1"
android:gravity="left" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/textView2"
android:layout_weight="1"
android:gravity="right" />
</LinearLayout>
</RelativeLayout>
4 Answers
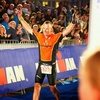
Steve Hunter
57,712 PointsHi there,
You want to bind the TextView called movieTitle
(so reference R.id.movieTitle
) and do this outside of onCreate
at the start of the class.
So, before onCreate
, add:
@BindView(R.id.movieTitle) TextView mTitleLabel;
I hope that helps,
Steve.

Kole Lleshaj
2,091 PointsBindView is not applicable as a local variable. Put "BindView" above onCreate method.
Like this:
public class MovieActivity extends Activity {
@BindView(R.id.movieTitle) TextView mTitleLabel; // code amended to correct error
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
}
}
[MOD: edited code block to make it display formatted & added comment to code - srh]
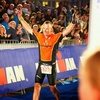
Steve Hunter
57,712 PointsYou need to bind the TextView
called movieTitle
- not the linearLayout
.

Kole Lleshaj
2,091 PointsYeah, you are totally right!
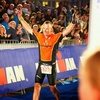
Steve Hunter
57,712 Points
Kristian Mazsar
3,936 PointsIt helped!! Thanks guys
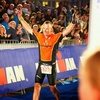
Steve Hunter
57,712 PointsNo problem! Glad to help.

Mohammed Amin
5,445 PointsSo you got the first part of three tasks correct. You've setup the class to be able to bind views by doing ButterKnife.bind(this);
However, the next two tasks ask's you to add member variables. You were trying to bind the view within the onCreate method which would yield in errors for you. Try to change where you are binding your variable to the class level instead. The third task is the same thing only you bind a different view.
Lastly, you may want to check the id you are using to bind the view. They ask you to bind the movie title text view and the ID for that is R.id.movieTItle not R.id.linearLayout. If you change this id to the correct id and move the variable to the class level, you should be able to complete the 2nd task. The third task if very similar to the second.
Here is an overview of how the MovieActivity should look;
public class MovieActivity extends Activity {
@BindView(R.id.movieTitle) TextView mTitleLabel;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
}
}