Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial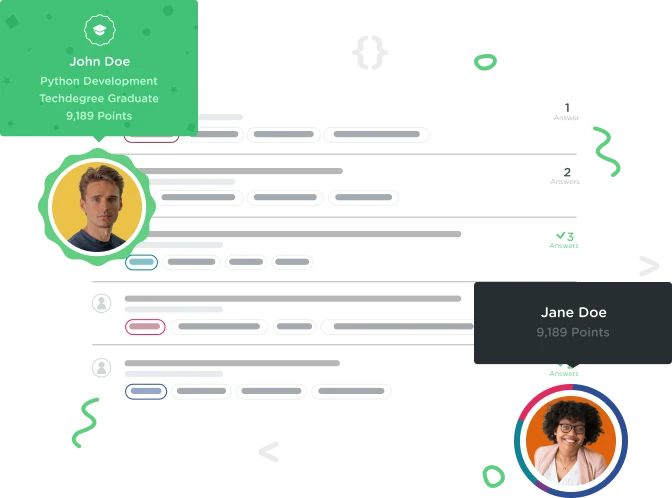

Nicholas Zavras
6,277 PointsCan't get it to end properly
Trying to get it to give the user two guesses: The initial guess, then one after a hint of higher or lower. When the player guesses higher, it hints lower, then hints higher (if the player didn't get it on their second try).
I understand this is because of the sequence of code, but I'm not sure how to make it skip to the 'end' after the player guesses wrong after a lower hint?
var correctGuess = false; var guess = prompt("Guess a number from 1 to 10!"); var random = Math.floor(Math.random() * 10) + 1;
if (parseInt(guess) === random) {correctGuess = true;} else if (parseInt(guess) > random) {var guessLower = prompt("Try lower!");} if (parseInt(guessLower) === random) {correctGuess = true;}
else if (parseInt(guess) < random); {var guessHigher = prompt("Try higher!");}
if (parseInt(guessHigher) === random) {correctGuess = true;}
if (correctGuess) {alert("You got it!");}
else {alert("Nope! The number was " + random + " !")}
4 Answers
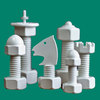
Steven Parker
229,783 PointsYou have a bit too much code .. you probably want something like this:
var correctGuess = false;
var guess = prompt("Guess a number from 1 to 10!");
var random = Math.floor(Math.random() * 10) + 1;
if (parseInt(guess) === random) {
correctGuess = true;
} else if (parseInt(guess) > random) {
guess = prompt("Try lower!");
correctGuess = parseInt(guess) === random;
} else {
guess = prompt("Try higher!");
correctGuess = parseInt(guess) === random;
}
if (correctGuess) {
alert("You got it!");
} else {
alert("Nope! The number was " + random + " !");
}
And when posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Nicholas Zavras
6,277 PointsThanks! I'm a little confused about setting the correctGuess from a value of 'false' to a value of 'parseInt(guess) === random;' as you did here:
} else if (parseInt(guess) > random) {
guess = prompt("Try lower!");
correctGuess = parseInt(guess) === random;
} else {
guess = prompt("Try higher!");
correctGuess = parseInt(guess) === random;
I'm also unsure why it's not still running the Try Higher part no matter what as mine is, is that because setting correctGuess to the user's guess equaling the random number causes it to skip down to the end of the code somehow?
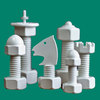
Steven Parker
229,783 PointsThe expression "parseInt(guess) === random
" compares the guess to the random number. If they are equal, the entire thing is the same as true
. Otherwise, its the same as false
.
I tested the revised version several times, and I got some "Try higher!" messages and also some "Try lower!" ones.

Nicholas Zavras
6,277 PointsAwesome appreciate the help

Omaid Royeen
Courses Plus Student 1,141 Points// set it to false var correctGuess= false;
var randomNumber = Math.floor(Math.random()*6)+1; var guess = prompt (" I am thinking of a number between 1 and 6.What is it?"); if(parseInt(guess)===randomNumber) { correctGuess=true; } else if( parseInt(guess) < randomNumber) { var guessMore = prompt("try again. the number I am thinking of is more than " + guess); if( parseInt(guessMore === randomNumber)){ correctGuess=true; } }
else if( parseInt(guess) > randomNumber) { var guessLess = prompt("try again. the number I am less than " + guess); if( parseInt(guessLess === randomNumber)) { correctGuess=true; } } if(correctGuess) { document.write("you are right"); } else { document.write("guess again you are wrong, the number was" + randomNumber + "!"); }