Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial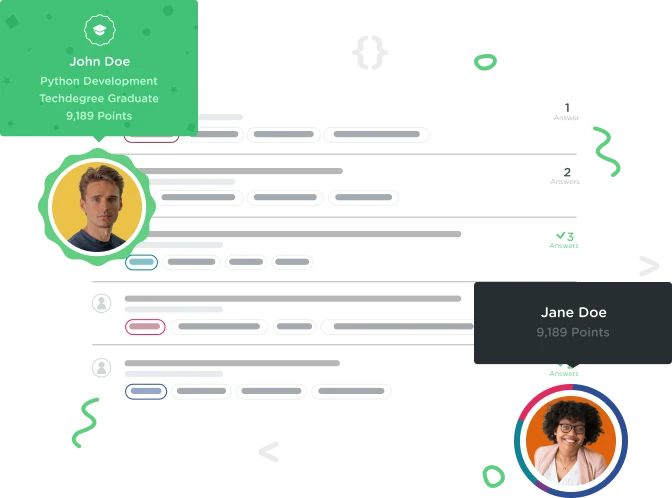

Leigh Maher
21,830 PointsCan't get it to work using the $.ajax() method
I'm trying to work out how you would write the code using the $.ajax() method instead of the $.getJSON() method, but I keep getting an error. Here's what I've done:
$(document).ready(function() {
// The user clicks on one of the animals in the list
$('button').on('click', function() {
// variable to hold the value of the items text
const animal = $(this).text();
$('button').removeClass('selected');
$(this).addClass('selected');
// Create the AJAX
$.ajax({
url: 'https://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?',
type: 'GET',
datType: 'json',
data: {
tags: animal,
format: 'json'
},
success: function(response) {
let statusHTML = `<ul>`;
$.each(response.items, function(i, picture) {
statusHTML += `<li class="grid-25 tablet-grid-50">`;
statusHTML += `<a href="${picture.link}" class="image">`;
statusHTML += `<img src="${picture.media.m}" class="thumbnail">`;
statusHTML += `</a></li>`;
})
statusHTML += `</ul>`;
$('#photos').html(statusHTML);
},
error: function (e, t) { // built in error message
alert(t);
}
}); // end ajax
});// end button click
}); // end redy
What am I doing wrong here?
Derrick Johnson
4,347 PointsDerrick Johnson
4,347 PointsYou just misspelled the
dataType
property:datType: 'json'
should be:
dataType: 'json'
Here's my code for reference: