Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial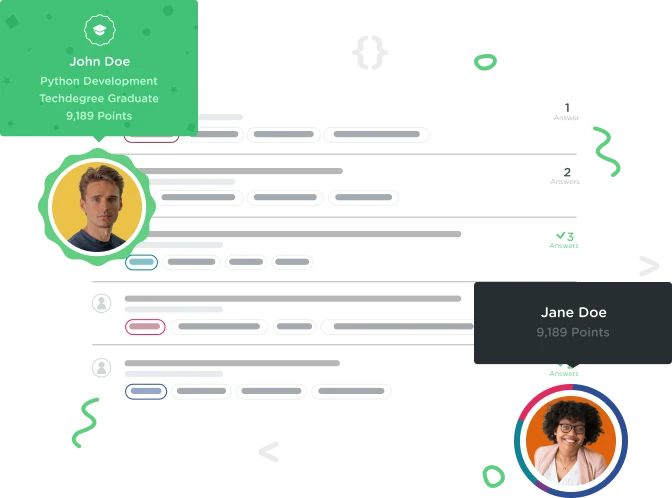

Yusuf Mohamed
2,631 PointsCan't get JSON data. I also get the error message even though I can't find any errors.
I've done everything like in the video but I can't get the JSON data to get to the logcat. I just get this message of {"code":400,"error":"The given location (or time) is invalid."}. I've also noticed another error java.io.FileNotFoundException but I don't know if this is anything major. I can't find the error that the message that we built pop up.
public class MainActivity extends AppCompatActivity {
private CurrentWeather mCurrentWeather;
public static final String TAG =MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "e33ba7eb325edad217b7c8b18e7e5889";
double latitude = 37.8267 ;
double longitude = -122.4233;
String forecastURL = "https://api.darksky.net/forecast/"+apiKey+"/"
+ latitude +" , "+ longitude+"";
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastURL)
.build();
//This is the request to get data from forecast.
okhttp3.Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(okhttp3.Call call, IOException e) {
}
@Override
public void onResponse(okhttp3.Call call , Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught", e);
}
catch (JSONException e)
{
Log.e(TAG, "Exception caught", e);
}
}
});
} else {
Toast.makeText(this, R.string.network_unavailable_message,
Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running!");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG ,"From JSON" + timezone);
return new CurrentWeather();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable =true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
public class CurrentWeather {
private String mIcon;
private long mTime;
private double mTemperature;
private double mHumidity;
private double mPrecipChance;
private String mSummary;
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public double getTemperature() {
return mTemperature;
}
public void setTemperature(double temperature) {
mTemperature = temperature;
}
public double getHumidity() {
return mHumidity;
}
public void setHumidity(double humidity) {
mHumidity = humidity;
}
public double getPrecipChance() {
return mPrecipChance;
}
public void setPrecipChance(double precipChance) {
mPrecipChance = precipChance;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
}
public class AlertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context)
.setTitle(R.string.error_title)
.setMessage(R.string.error_message)
.setPositiveButton(R.string.error_ok_button_text, null);
AlertDialog dialog = builder.create();
return dialog;
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.mohamed.stormy">
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
</application>
</manifest>
'
01-07 01:39:47.758 6523-6523/? W/ViewRootImpl[MainActivity]: Dropping event due to root view being removed: MotionEvent { action=ACTION_MOVE, actionButton=0, id[0]=0, x[0]=76.0, y[0]=-589.0, toolType[0]=TOOL_TYPE_FINGER, buttonState=0, metaState=0, flags=0x0, edgeFlags=0x0, pointerCount=1, historySize=2, eventTime=44539337, downTime=44539232, deviceId=8, source=0x1002 }
01-07 01:39:47.758 6523-6523/? W/ViewRootImpl[MainActivity]: Dropping event due to root view being removed: MotionEvent { action=ACTION_UP, actionButton=0, id[0]=0, x[0]=76.0, y[0]=-589.0, toolType[0]=TOOL_TYPE_FINGER, buttonState=0, metaState=0, flags=0x0, edgeFlags=0x0, pointerCount=1, historySize=0, eventTime=44539345, downTime=44539232, deviceId=8, source=0x1002 }
01-07 16:36:16.273 802-13762/? I/ActivityManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10000000 cmp=com.example.mohamed.stormy/.MainActivity} from uid 2000
01-07 16:36:16.398 802-13761/? I/ActivityManager: Start proc 31868:com.example.mohamed.stormy/u0a150 for activity com.example.mohamed.stormy/.MainActivity
01-07 17:29:43.352 802-4254/? I/ActivityManager: Force finishing activity ActivityRecord{539cb9a u0 com.example.mohamed.stormy/.MainActivity t15747}
01-07 17:29:43.363 802-4254/? I/ActivityManager: Force finishing activity ActivityRecord{539cb9a u0 com.example.mohamed.stormy/.MainActivity t15747 f}
01-07 17:29:43.363 802-4254/? W/ActivityManager: Duplicate finish request for ActivityRecord{539cb9a u0 com.example.mohamed.stormy/.MainActivity t15747 f}
01-07 17:30:00.460 802-15442/? I/ActivityManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10000000 cmp=com.example.mohamed.stormy/.MainActivity} from uid 2000
01-07 17:30:00.660 802-2953/? I/ActivityManager: Start proc 2270:com.example.mohamed.stormy/u0a150 for activity com.example.mohamed.stormy/.MainActivity
01-07 17:30:39.630 802-15441/? W/ActivityManager: Force removing ActivityRecord{713aed5 u0 com.example.mohamed.stormy/.MainActivity t15748}: app died, no saved state
01-07 17:30:39.721 802-4269/? I/WindowManager: WIN DEATH: Window{a166d7d u0 com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity}
01-07 17:30:44.553 802-13765/? I/ActivityManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10000000 cmp=com.example.mohamed.stormy/.MainActivity} from uid 2000
01-07 17:30:44.583 802-13760/? I/ActivityManager: Start proc 2704:com.example.mohamed.stormy/u0a150 for activity com.example.mohamed.stormy/.MainActivity
01-07 17:31:10.288 802-15439/? W/ActivityManager: Force removing ActivityRecord{191380e u0 com.example.mohamed.stormy/.MainActivity t15749}: app died, no saved state
01-07 17:31:10.364 802-814/? I/WindowManager: WIN DEATH: Window{12ed6a5 u0 com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity}
01-07 17:31:19.483 802-2841/? I/ActivityManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10000000 cmp=com.example.mohamed.stormy/.MainActivity} from uid 2000
01-07 17:31:19.515 802-15437/? I/ActivityManager: Start proc 2834:com.example.mohamed.stormy/u0a150 for activity com.example.mohamed.stormy/.MainActivity
01-07 17:31:19.931 2834-2834/? D/MainActivity: Main UI code is running!
01-07 17:31:21.453 2834-2868/? V/MainActivity: {"code":400,"error":"The given location (or time) is invalid."}
01-07 17:31:21.557 428-428/? D/SurfaceFlinger: duplicate layer name: changing com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity to com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity#1
01-07 17:31:43.483 2834-2834/? W/ViewRootImpl[MainActivity]: Dropping event due to root view being removed: MotionEvent { action=ACTION_MOVE, actionButton=0, id[0]=0, x[0]=224.0, y[0]=-573.0, toolType[0]=TOOL_TYPE_FINGER, buttonState=0, metaState=0, flags=0x0, edgeFlags=0x0, pointerCount=1, historySize=2, eventTime=74158292, downTime=74158267, deviceId=8, source=0x1002 }
01-07 17:31:56.184 802-13762/? W/ActivityManager: Force removing ActivityRecord{fb981cb u0 com.example.mohamed.stormy/.MainActivity t15750}: app died, no saved state
01-07 17:31:56.191 802-13762/? I/WindowManager: Failed to capture screenshot of Token{58a4da8 ActivityRecord{fb981cb u0 com.example.mohamed.stormy/.MainActivity t15750 f}} appWin=Window{e62aee u0 com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity} drawState=4
01-07 17:39:08.779 802-2736/? I/ActivityManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10000000 cmp=com.example.mohamed.stormy/.MainActivity} from uid 2000
01-07 17:39:08.845 802-4281/? I/ActivityManager: Start proc 5110:com.example.mohamed.stormy/u0a150 for activity com.example.mohamed.stormy/.MainActivity
01-07 17:39:09.286 5110-5110/com.example.mohamed.stormy D/MainActivity: Main UI code is running!
01-07 17:39:09.689 802-827/? I/ActivityManager: Displayed com.example.mohamed.stormy/.MainActivity: +860ms (total +6m54s177ms)
01-07 17:39:09.978 5110-5127/com.example.mohamed.stormy V/MainActivity: {"code":400,"error":"The given location (or time) is invalid."}
01-07 17:39:10.135 428-428/? D/SurfaceFlinger: duplicate layer name: changing com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity to com.example.mohamed.stormy/com.example.mohamed.stormy.MainActivity#1
01-07 17:39:13.407 5143-5192/? E/Nova.IconTheme: website.leifs.delta item tag must specify component, drawable, found: { component=ComponentInfo{com.nytimes.android/com.nytimes.android.activity.MainActivity_}, name=ny_times, } Check your spelling
01-07 17:39:13.407 5143-5192/? E/Nova.IconTheme: website.leifs.delta item tag must specify component, drawable, found: { component=ComponentInfo{com.nytimes.android/com.nytimes.android.MainActivity}, name=ny_times, } Check your spelling
01-07 17:39:14.134 5143-5192/? E/Nova.IconTheme: website.leifs.delta item tag must specify component, drawable, found: { component=ComponentInfo{de.lineas.lit.ntv.android/de.lineas.ntv.tablet.MainActivity}, name=n_tv, } Check your spelling
01-14 17:37:12.576 1651-1665/system_process I/ActivityManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10000000 cmp=com.example.mohamed.stormy/.MainActivity} from uid 0 on display 0
01-14 17:37:12.951 1651-1797/system_process I/ActivityManager: Start proc 3299:com.example.mohamed.stormy/u0a33 for activity com.example.mohamed.stormy/.MainActivity
01-14 17:37:15.112 3299-3299/com.example.mohamed.stormy D/MainActivity: Main UI code is running!
01-14 17:37:15.800 3299-3348/com.example.mohamed.stormy V/MainActivity: {"code":400,"error":"The given location (or time) is invalid."}
01-14 17:37:16.215 1651-1674/system_process I/ActivityManager: Displayed com.example.mohamed.stormy/.MainActivity: +3s276ms
1 Answer

Seth Kroger
56,414 PointsWhen you're building the forecast URL try removing the spaces around the comma.
Yusuf Mohamed
2,631 PointsYusuf Mohamed
2,631 PointsThank you very much! :)