Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial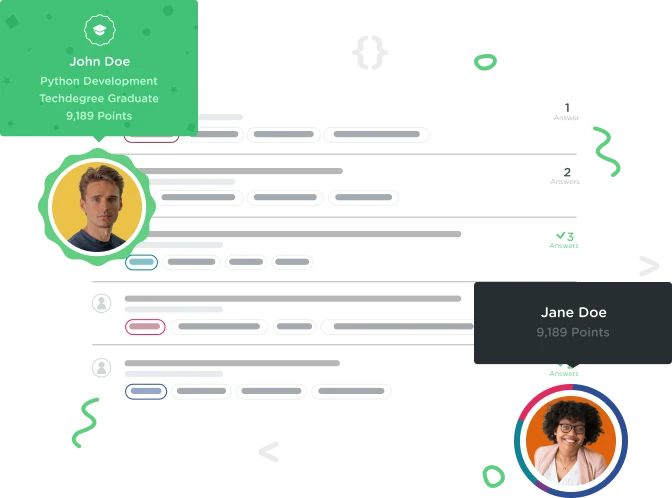

Hasan Nagaria
4,855 PointsCant get my custom adapter/list to show
I have tried EVERYTHING, Im sure I have gone back and checked my code three times line for line. When I hit my daily button all I get is that there is no data to display the app doesnt crash or give me any errors.
I went through all the questions and a popular problem people had was setting the adapter and I have done that too. Im really bummed because I cant progress for three days now.
20 Answers

maivandahmadzai
3,467 PointsNo worries found it in another thread. The teacher forgot to add setListAdapter(dayAdapter); to set the adapter after creating the new adapter in the onCreate method of DailyForecastActivity.
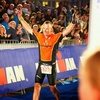
Steve Hunter
57,712 PointsGlad you fixed it!
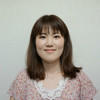
Ailing Cui
3,849 PointsThank you
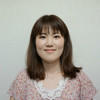
Ailing Cui
3,849 PointsThank you

Nicholas Gorman
1,718 PointsI ran back through the code... like super far back when we started creating the DayAdapter. Go to the DayAdapter.java file and look at the implemented getCount() method. My listview wasn't showing anything either, it seems I forgot to add in the count number. Try putting this in... @Override public int getCount() { return mDays.length; }
make sure to replace the original override 0, with mDays.length;
If the app crashes after you add that line in, delete the entire method and reimplement it. That should do the trick =)
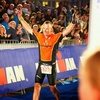
Steve Hunter
57,712 PointsHi Hasan,
Does your current forecast load?
What do you get when you click the 7-day button?
Can you post your code from Day
where the Parcelable
interface is implemented. The error may be in there, perhaps.
Steve.
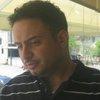
Mazen Halawi
7,806 Pointsnot sure the use of the layout you are using in the ListActivity and if its covering the whole activity for you. can you remove that line and try to compile it again. if its still not showing, paste the code of your DayAdapter class.

Hasan Nagaria
4,855 PointsAt the moment I get a orange gradient screen showing "There is no data to display" This is the situation we handled when there was no data being recieved. However on the main screen i do get the current temperature and time perfectly.
When I remove the layout as you said then I get a white back ground.

Hasan Nagaria
4,855 Pointspackage com.example.administrator.stormy.weather;
import android.os.Parcel;
import android.os.Parcelable;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
/**
* Created by Administrator on 1/9/2016.
*/
public class Day implements Parcelable{
private long mTime;
private String mSummary;
private double mTemperatureMax;
private String mIcon;
private String mTimezone;
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperatureMax() {
return (int) Math.round(mTemperatureMax);
}
public void setTemperatureMax(double temperatureMax) {
mTemperatureMax = temperatureMax;
}
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public int getIconId(){
return Forecast.getIconId(mIcon);
}
public String getDayOfTheWeek(){
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(mTimezone));
Date dateTime = new Date(mTime * 1000);
return formatter.format(dateTime);
}
//anytime we implement an interface we need to implement the methods of that interface.
//we will leave describeContents alone as we dont need it.
@Override
public int describeContents() {
return 0;
}
//here we will write the data into the destination parcel...this is the wrapping part.
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeString(mSummary);
dest.writeDouble(mTemperatureMax);
dest.writeString(mIcon);
dest.writeString(mTimezone);
}
//now we need to write the code to unwrap it as well...
//to unwrap it we will use a private constructor which takes the parcel object as a parameter.
private Day(Parcel in){
mTime = in.readLong();
mSummary = in.readString();
mTemperatureMax = in.readDouble();
mIcon = in.readString();
mTimezone = in.readString();
}
public Day(){}
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
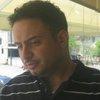
Mazen Halawi
7,806 Pointscan you paste the DayAapter code

Hasan Nagaria
4,855 Pointspublic class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0;//we will not use this. Tag items for easy reference.
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null){
//brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel= (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else{
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
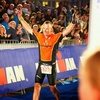
Steve Hunter
57,712 PointsWhere is your ViewHolder
class defined? In my code it is a static class within DayAdapter
. You must have defined it somewhere; I just can't see it.
Steve.
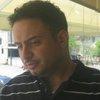
Mazen Halawi
7,806 Pointsreplace this line convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
with this: convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, parent, false);
and comment out the if statement, its not needed.
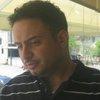
Mazen Halawi
7,806 PointsCan you try this code and comment yours out
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
LayoutInflater inflater = (LayoutInflater)mContext.getSystemService(mContext.LAYOUT_INFLATER_SERVICE);
View view = inflater.inflate(R.layout.daily_list_item, parent, false);
Day day = mDays[position];
((ImageView)view.findViewById(R.id.iconImageView)).setImageResource(day.getIconId());
((TextView)view.findViewById(R.id.temperatureLabel)).setText(day.getTemperatureMax() + “”);
((TextView)view.findViewById(R.id.dayNameLabel)).setText(day.getDayOfTheWeek());
return view;
}
[MOD: edited code block]
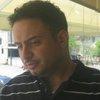
Mazen Halawi
7,806 Pointsalso im not sure how the layout is but usually the listActivity shouldnt be covered with another layout so keep the setContentView commented out for now and try

Hasan Nagaria
4,855 PointsThanks for the reply. I still get nothing. when I copy pasted your code, I got the same screen which warns that no data is available. When I comment out the layout i get a white background and nothing else.
I was fine upuntil ben made the custom list and adapteers and since then i have traced his code three times and i cant seem to make my custom adpater/list work im really not sure what im missing.
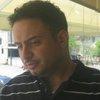
Mazen Halawi
7,806 Pointsbefore you initialize the DayAdapter adapter.... can you Log.v("DEBUG", String.valueOf(mDays.length));

Hasan Nagaria
4,855 Pointspackage com.example.administrator.stormy.adapters;
import android.content.Context;
import android.media.Image;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.administrator.stormy.R;
import com.example.administrator.stormy.weather.Day;
import org.w3c.dom.Text;
/**
* Created by Administrator on 1/16/2016.
*/
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0;//we will not use this. Tag items for easy reference.
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null){
//brand new
//convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, parent, false);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel= (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else{
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
//view holder class will hold the views that are added to the list layout
private static class ViewHolder{
ImageView iconImageView;
TextView temperatureLabel;
TextView dayLabel;
}
}
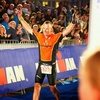
Steve Hunter
57,712 PointsThank you - just checking! :-)

Hasan Nagaria
4,855 Pointswhen I log it i get this:
01-23 22:53:26.158 29575-29575/com.example.administrator.stormy V/DEBUG﹕ 0
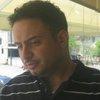
Mazen Halawi
7,806 Pointsthat means there is nothing wrong, the array you are sending is empty
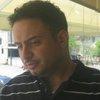
Mazen Halawi
7,806 Pointstest by sending a custom array like String[] arr = {"apple", "orange", "banana", lemon"} and see what you get.

Hasan Nagaria
4,855 Pointsalright give me a second, Ill just do that.

maivandahmadzai
3,467 PointsI have the same problem.. did you solve it?

maivandahmadzai
3,467 PointsI have 8 in the array,

Nicholas Gorman
1,718 PointsDid you ever solve this problem? I seem to be running into the same thing
Hasan Nagaria
4,855 PointsHasan Nagaria
4,855 Points