Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial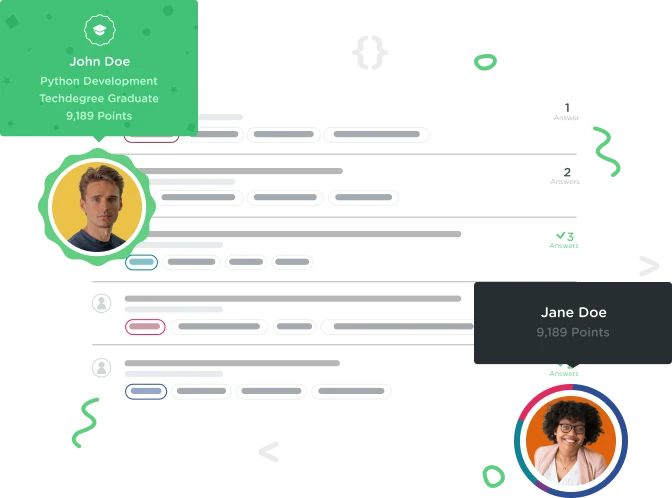

Tayler Kemsley
21,479 PointsCan't get my while loop to stop looping!
Hi guys,
So I'm a beginner in Python, and I'm at the part of the lesson where you're asked to make a basic shopping list.
I thought I'd give it a try on my own before I tried the lesson to make sure I was getting a good idea of the language but am struggling. Here's my code so far:
shopping_list = []
finished = False
def add_item(item):
if item.upper() == "DONE":
print (shopping_list)
finished = True
elif item in shopping_list:
print ("This item is already on your list")
else:
shopping_list.append(item)
while finished == False:
add_item(input("What would you like to add?: "))
It works fine, but I can't get the loop to STOP looping. As you can see in the add_items function, the finished variable should change to True and stop the loop but it never does.
I figure I'll never learn if I don't ask so... here I am! Thanks in advance guys.
2 Answers
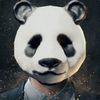
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi Tayler, I have taken a look at your code and found that you have not used the global
keyword inside your function. This is required if you want to change values of variables outside of the function. Inside your function, at the top, add global
followed by the name of the variable you want to change. Your code should look like this:
shopping_list = []
finished = False
def add_item(item):
global finished #add this line in
if item.upper() == "DONE":
print (shopping_list)
finished = True
elif item in shopping_list:
print ("This item is already on your list")
else:
shopping_list.append(item)
while finished == False:
add_item(input("What would you like to add?: "))
This is something you may not have learned but it is needed to change variables which are outside the function.
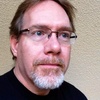
Chris Freeman
Treehouse Moderator 68,423 PointsThis a great question. It is important to know when a variable is referencing a local variable or a global (module) variable.
When referencing a variable, if not found in the local scope of a function, the next level up is checked for the variable. This continues until the module level.
The exception is when a variable is assigned, the variable becomes local.
shopping_list = []
finished = False # global finished
def add_item(item):
if item.upper() == "DONE":
print (shopping_list)
finished = True. # local finished
elif item in shopping_list:
print ("This item is already on your list")
else:
shopping_list.append(item)
while finished == False: # global finished
add_item(input("What would you like to add?: "))
To make the finished
inside of add_item
behave as you wish, add a global
statement:
def add_item(item):
global finished
if item.upper() == "DONE":
print (shopping_list)
finished = True. # global finished
elif item in shopping_list:
print ("This item is already on your list")
else:
shopping_list.append(item)
Tayler Kemsley
21,479 PointsTayler Kemsley
21,479 PointsAh that's interesting! Thanks so much.
So the "finished" variable inside the add_item function was ONLY inside that function.
I was probably jumping the gun, I'd wager the next video I watch will explain this!
Thanks again.