Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial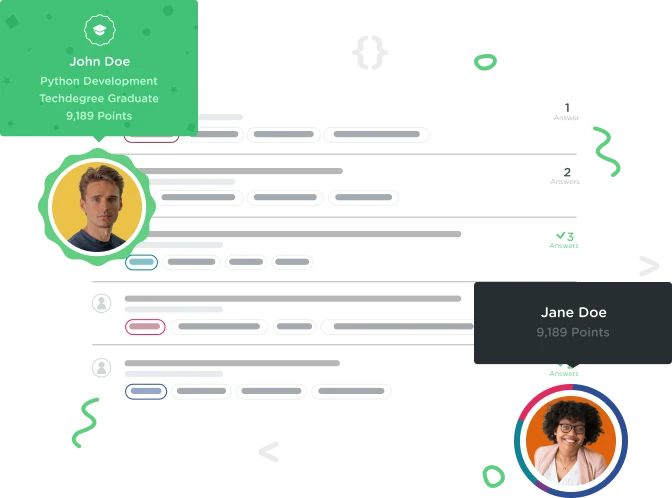

Chris Collier
17,774 PointsCan't get onclick event attached to add button/firing correctly
Hi, here's my code:
//Problem: User interaction doesn't provide desired results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//Add a new task
var addTask = function() {
console.log("Add task...");
alert("Add task");
//When the button is pressed
//Create a new list item with teh text from #new-task:
//Input (checkbox)
//Label
//Input (text)
//Button.edit
//Button.delete
//Each element needs modified and appended
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...");
//When the Edit button is pressed
//If the class of the parent is .editMode
//Switch from .editMode
//Label text become the input's value
//else
//Switch to .editMode
//Input value become's the label's text
//Toggle .editMode on the parent
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
//When the Delete button is pressed
//Remove the parent list item from the ul
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Task complete...");
//When the Checkbox is checked
//Append the task list item to the #completed-tasks
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//When the Checkbox is unchecked
//Append the task list item to the #incomplete-tasks
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
I believe I've copied it very well from what is in the videos. When I click the addButton, there's supposed to be a console.log "Add task...", but it isn't working. I tried adding an alert(), but no dice there either.
In the console, addButton correctly refers to the HTML:
<button>Add</button>
However, addButton.onclick returns null. Does anyone know what I'm doing wrong?
4 Answers

Austin Whipple
29,725 PointsYou may need to invoke the function with the parenthesis:
addButton.onclick = addTask();
Could also give using an event listener a try:
addButton.addEventListener("click", addTask());

Chris Collier
17,774 PointsThanks I tried addEventListener and when that didn't work, I knew something was systemically wrong. It turns out that, for some reason, my browser wasn't loading the most recent .js file from Workspace. I had to clear my cache to fix.

Jimmy Brannon
3,566 PointsI'm having the same issue in both chrome and firefox.
The two work arounds I've found is using:
addButton.addEventListener("click", addTask);
or
add an ID to the button and changing getElementsByTagName to getElementById
I still want to know why it works in the video and not in my browser (new version perhaps?)
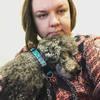
Bonnie Martin
13,671 PointsI had the same problem. Adding parentheses worked, like: addButton.onClick = addTask();
Does anyone know why that would be necessary? What could have changed with JavaScript or Chrome since this video was made that would make that necessary? Just curious.

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsStrangely, the opposite worked for me - I removed the parenthesis and it worked lol.