Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial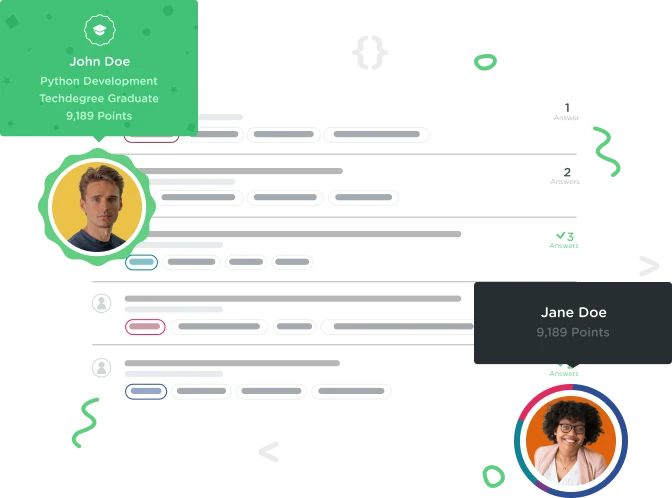

Laura Mckay
599 PointsCan't get part of my code to run!
Have followed everything and somehow part of my code doesn't run! Have spent ages on this and driving me mad! About ready to give up! Won't run "Dispenser is full" or any of the "Yum" or "Chomp" lines. Please help!
Sorry if I'm being stupid!
EXAMPLE CLASS: /**
-
Created by Lawz on 17/03/2017. */ public class Example {
public static void main(String[] args) { // Your amazing code goes here... System.out.println("We are making a new PEZ Dispenser"); System.out.printf("FUN FACT: There are %d pez in every pez dispenser %n", PezDispenser.MAX_PEZ);
PezDispenser dispenser = new PezDispenser("Darth Vader"); System.out.printf("The dispensener is %s %n", dispenser.getCharacterName()); if (dispenser.isEmpty()) { System.out.println("Dispenser is empty"); } System.out.println("Filling the dispenser with delicious PEZ..."); dispenser.fill(); if (!dispenser.isEmpty()) { System.out.printf("Dispenser is full"); } while (dispenser.dispense()) { System.out.printf("YUM"); } if (dispenser.isEmpty()) { System.out.println("You ate all the PEZ!"); } dispenser.fill(4); dispenser.fill(2); while (dispenser.dispense()) { System.out.printf("Chomp chomp!!"); } try { dispenser.fill(400); System.out.printf("This will never happen"); } catch (IllegalArgumentException iae) { System.out.println("Whoa there!"); System.out.printf("The error was %s", iae.getMessage()); }
} }
PEZDISPENSER CLASS: /**
-
Created by Lawz on 17/03/2017. */ class PezDispenser { private String characterName; public static final int MAX_PEZ = 12; private int pezCount;
public PezDispenser(String characterName) { this.characterName = characterName; pezCount = 0; }
public void fill() { fill(MAX_PEZ); }
public void fill(int pezAmount) { int newAmount = pezCount + pezAmount; if(newAmount > MAX_PEZ) { throw new IllegalArgumentException("Too many PEZ!!!"); } }
public boolean dispense() { boolean wasDispensed = false; if(!isEmpty()) { pezCount--; wasDispensed = true; } return wasDispensed; }
public boolean isEmpty() { return pezCount == 0; }
public String getCharacterName() { return characterName; } }
1 Answer

Seth Kroger
56,413 PointsIn your fill(amount) method you forgot to set the amount of PEZ to newAmount at the end (after you check for overfilling). Because of this your dispenser is always empty.
Ilya Magomedov
1,717 PointsIlya Magomedov
1,717 PointsHi Laura,
try to change this line of code (while (dispenser.dispense()) { System.out.printf("YUM");)
to
while (!dispenser.dispense()) { System.out.println("YUM");
hope it helps)