Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial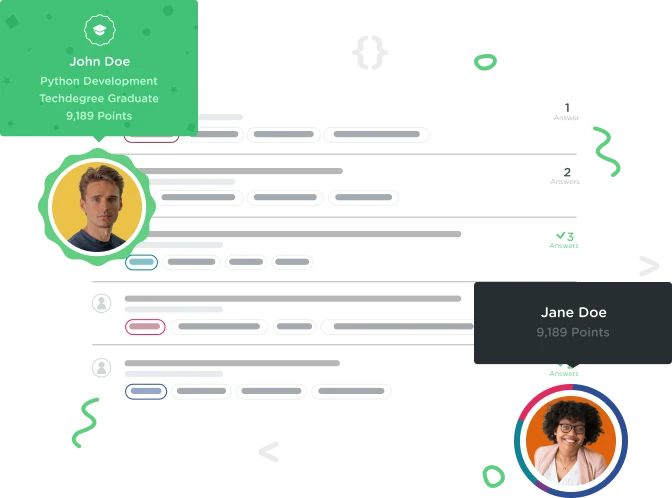

Tom Dalton
1,793 PointsCan't get past part 4, unhelpful error
I get up to step 3, but at step 4 (add the serialiser.Serialize
line), I keep getting an error: " Bummer! Did you call the Serialize method on the 'serializer' object?". Well, I'm pretty sure I have called it, so the error isn't really helpful. I followed through the player example for the course and have pretty much exactly the same code for the football player serialisation, and that works, so I'm wondering if this is a but with the course itself or the test framework Treehouse is using?!
public static void SerializeWeatherForecasts(List<WeatherForecast> weatherForecasts, string fileName)
{
var serialiser = new JsonSerializer();
using (var writer = new StreamWriter(fileName))
using (var jsonWriter = new JsonTextWriter(writer))
{
serialiser.Serialize(jsonWriter, weatherForecasts);
}
}
using System;
using System.IO;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
double precipitation;
if (double.TryParse(values[4], out precipitation))
{
weatherForecast.PrecipitationChance = precipitation;
}
if (double.TryParse(values[5], out precipitation))
{
weatherForecast.PrecipitationAmount = precipitation;
}
return weatherForecast;
}
public static List<WeatherForecast> DeserializeWeather(string fileName)
{
var weatherForecasts = new List<WeatherForecast>();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
var serializer = new JsonSerializer();
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}
return weatherForecasts;
}
public static void SerializeWeatherForecasts(List<WeatherForecast> weatherForecasts, string fileName)
{
var serialiser = new JsonSerializer();
using (var writer = new StreamWriter(fileName))
using (var jsonWriter = new JsonTextWriter(writer))
{
serialiser.Serialize(jsonWriter, weatherForecasts);
}
}
}
}
using System;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
[JsonProperty(PropertyName = "weather_station_id")]
public string WeatherStationId { get; set; }
[JsonProperty(PropertyName = "time_of_day")]
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
public int Temperature { get; set; }
[JsonProperty(PropertyName = "precipitation_chance")]
public double PrecipitationChance { get; set; }
[JsonProperty(PropertyName = "precipitation_amount")]
public double PrecipitationAmount { get; set; }
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
2 Answers
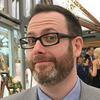
Simon McGuirk
2,673 PointsThink its because you've named the var serialiser
and not serializer
which is what the code checker may be looking for.
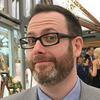
Simon McGuirk
2,673 PointsNo worries. Glad it helped :)
Tom Dalton
1,793 PointsTom Dalton
1,793 PointsThanks! I added that variable at part 2 and it accepted it then, so didn't think it would be a spelling issue! Thanks very much for the extra pair of eyes!