Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial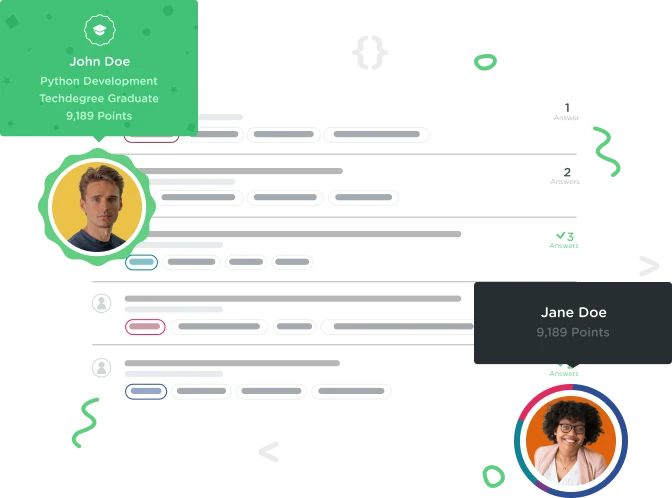
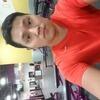
Tommy Choe
38,156 PointsCan't get sprites from the sprites.atlas folder to show up.
I'm trying to code the "Space Cat" game in Swift rather than Objective-C. I can't get the "machine_1" image from the Sprites.atlas to show up no matter what I do. I've checked the build phases tab and the Sprites.atlas folder is listed as a resource.
Here's my code: TitleScene:
//
// TitleScene.swift
// Space Cat
//
// Created by Tommy Choe on 11/3/15.
// Copyright © 2015 Tommy Choe. All rights reserved.
//
import SpriteKit
class TitleScene: SKScene {
override func didMoveToView(view: SKView) {
/* Setup your scene here */
let backgroundImage = SKSpriteNode(imageNamed: "splash_1")
backgroundImage.position = CGPointMake(CGRectGetMidX(self.frame), CGRectGetMidY(self.frame))
backgroundImage.size = self.frame.size
self.addChild(backgroundImage)
}
override func touchesBegan(touches: Set<UITouch>, withEvent event: UIEvent?) {
let gameScene = GameScene()
let transition = SKTransition.fadeWithDuration(1.0)
self.view?.presentScene(gameScene, transition: transition)
}
}
GameScene
//
// GameScene.swift
// Space Cat
//
// Created by Tommy Choe on 11/3/15.
// Copyright (c) 2015 Tommy Choe. All rights reserved.
//
import SpriteKit
class GameScene: SKScene {
override func didMoveToView(view: SKView) {
/* Setup your scene here */
//background image
let backgroundImage = SKSpriteNode(imageNamed: "background_1")
backgroundImage.position = CGPointMake(CGRectGetMidX(self.frame), CGRectGetMidY(self.frame))
backgroundImage.size = self.frame.size
self.addChild(backgroundImage)
//machine
let texture = SKTexture(imageNamed: "machine_1")
let machine = SKSpriteNode(texture: texture)
machine.position = CGPointMake(CGRectGetMidX(self.frame), CGRectGetMidY(self.frame))
self.addChild(machine)
}
}
GameViewController
//
// GameViewController.swift
// Space Cat
//
// Created by Tommy Choe on 11/3/15.
// Copyright (c) 2015 Tommy Choe. All rights reserved.
//
import UIKit
import SpriteKit
class GameViewController: UIViewController {
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
if let scene = TitleScene(fileNamed:"TitleScene") {
// Configure the view.
let skView = self.view as! SKView
skView.showsFPS = true
skView.showsNodeCount = true
/* Sprite Kit applies additional optimizations to improve rendering performance */
skView.ignoresSiblingOrder = true
/* Set the scale mode to scale to fit the window */
scene.scaleMode = .ResizeFill
skView.presentScene(scene)
}
}
override func shouldAutorotate() -> Bool {
return true
}
override func supportedInterfaceOrientations() -> UIInterfaceOrientationMask {
if UIDevice.currentDevice().userInterfaceIdiom == .Phone {
return .AllButUpsideDown
} else {
return .All
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Release any cached data, images, etc that aren't in use.
}
override func prefersStatusBarHidden() -> Bool {
return true
}
}
Any ideas on what could be wrong Amit Bijlani or Pasan Premaratne? Any help would be appreciated.
1 Answer
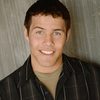
Kyler Smith
10,110 PointsTommy! I know this was a while ago and I want to know if you ever finished it in Swift? I am doing the same thing and made a struct for MachineNode and created a method named getMachine(position: CGPoint){} that would return the machine at the correct position. I am stuck a little bit further down and have some questions if you ended up finishing this in Swift.
import SpriteKit
import Foundation
import UIKit
struct MachineNode {
let texturesArray = [SKTexture(imageNamed: "machine_1"),
SKTexture(imageNamed: "machine_2")]
func getMachine(view: SKScene) -> SKSpriteNode {
let machine = SKSpriteNode(imageNamed: "machine_1")
machine.position = CGPoint(x: CGRectGetMidX(view.frame), y: 65)
let machineAnimation = SKAction.animateWithTextures(self.texturesArray, timePerFrame: 0.4)
let machineRepeat = SKAction.repeatActionForever(machineAnimation)
machine.runAction(machineRepeat)
return machine
}
}
This is my gameplay scene, where i call the machine.
//
// GamePlayScene.swift
// Space Cat Swift
//
// Created by Kyler on 7/21/16.
// Copyright © 2016 Kyler. All rights reserved.
//
import SpriteKit
import UIKit
import Foundation
class GamePlayScene: SKScene {
override func didMoveToView(view: SKView) {
let background = SKSpriteNode(imageNamed: "background_1")
background.position = CGPoint(x: CGRectGetMidX(self.frame), y: CGRectGetMidY(self.frame))
background.size = CGSizeMake(CGRectGetMaxX(self.frame), CGRectGetMaxY(self.frame))
self.addChild(background)
// Call the struct and create and display it
let machine: MachineNode = MachineNode()
self.addChild(machine.getMachine(self))
}
}