Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial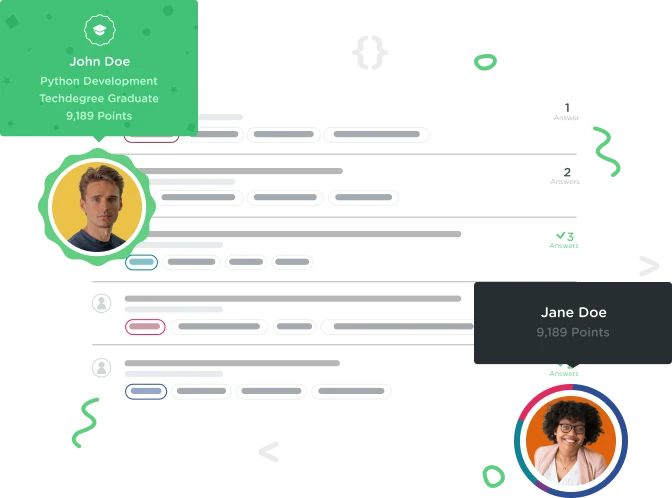
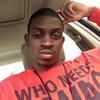
Eric Kelly
Courses Plus Student 1,555 PointsCan't get the frog to move, even though the code is correct and connected to the prefab itself.
using UnityEngine; using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidbody;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
playerRigidbody = GetComponent<Rigidbody> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void fixedUpdate () {
if (movement != Vector3.zero) {
Quaternion targetRotation = Quaternion.LookRotation (movement, Vector3.up);
Quaternion newRotation = Quaternion.Lerp (playerRigidbody.rotation, targetRotation, turningSpeed * Time.deltaTime);
playerRigidbody.MoveRotation (newRotation);
playerAnimator.SetFloat ("Speed", 3f);
} else {
playerAnimator.SetFloat ("Speed", 0f);
}
}
}
2 Answers
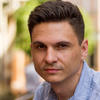
Nick Pettit
Treehouse TeacherHi Eric,
You wrote "fixedUpdate ()" instead of "FixedUpdate ()". Notice the capital F, as C# is a case-sensitive language.
Your code editor probably didn't call this out as an error because it thinks you're defining a function, rather than attempting to execute code when the "FixedUpdate ()" message is sent in the Unity engine.

Thomas Lian Ødegaard
9,540 PointsGo to Edit --> Project Settings --> Input. And check if the horizontal and vertical axe got the up and down, left and right button. It should be under Negative button. Example on horizontal the negative button should be left.
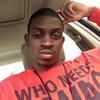
Eric Kelly
Courses Plus Student 1,555 PointsThe project settings are correct. From what I see, it looks as if my player movement script is not enabled. In the video underneath Player Movement (script), mine seems to be faded like its disabled, unlike in the video. Could this be the issue?
Eric Kelly
Courses Plus Student 1,555 PointsEric Kelly
Courses Plus Student 1,555 PointsThanks a million Nick. Can't believe I missed that.