Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial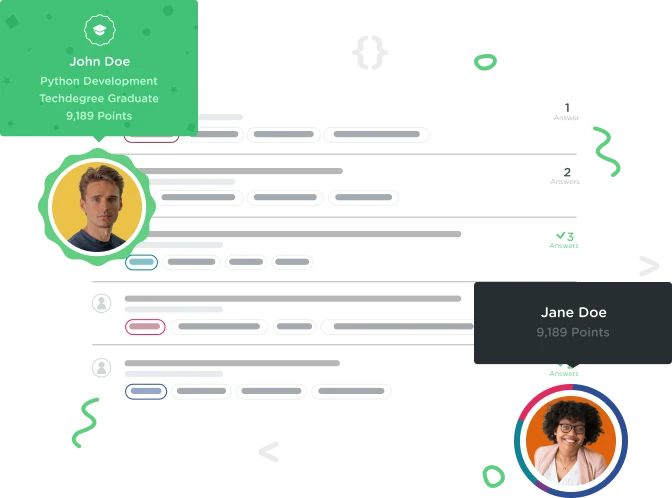

Casey Beaver
5,619 PointsCan't get the RGB to populate correctly in the html. So no background color is coming through. Can someone help?
I have two logs inside my code that is showing me that my RGB is being generated, but the html isn't recognizing it as all of my backgrounds are white. What am I doing wrong?
let [red, green, blue] = [0]; let randomRGB; let html = ''; const main = document.querySelector('main');
function getNumber() { return Math.floor(Math.random() * 256); }
red = getNumber(); green = getNumber(); blue = getNumber(); console.log(red, green, blue);
for (i = 0; i <= 10; i++) {
let randomRGB = rgb( ${red}, ${green}, ${blue} )
console.log(randomRGB);
html += <div style=background-color: ${randomRGB}">${i}</div>
;
}
main.innerHTML = html;
6 Answers
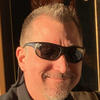
Peter Vann
36,425 PointsHi Casey!
BTW, for testing you can paste this code:
<!DOCTYPE html>
<html>
<body>
<main></main>
<script>
//let [red, green, blue] = [0];
let randomRGB;
let html = '';
const main = document.querySelector('main');
function getNumber() {
return Math.floor(Math.random() * 256);
}
for (i = 0; i <= 10; i++) {
let red = getNumber();
let green = getNumber();
let blue = getNumber();
console.log(red, green, blue);
let randomRGB = `rgb(${red}, ${green}, ${blue})`;
console.log(randomRGB);
html += `<div style="background-color: ${randomRGB}">${i}</div>`;
}
main.innerHTML = html;
</script>
</body>
</html>
Into the left pane here (completely overwrite what's there) and run it:
https://www.w3schools.com/js/tryit.asp?filename=tryjs_array
More info (Markdown):
https://github.com/treehouse/cheatsheets/blob/master/markdown_basics/cheatsheet.md
https://teamtreehouse.com/community/howto-guide-markdown-within-posts
I hope that helps.
Stay safe and happy coding!
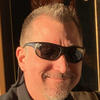
Peter Vann
36,425 PointsHi Casey!
One issue I see right off the bat is your template literal is missing backticks.
This:
let randomRGB = rgb( ${red}, ${green}, ${blue} )
Should be:
let randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
That should get you further along, anyhow...
I hope that helps.
Stay safe and happy coding!

Casey Beaver
5,619 PointsHi Peter,
Thanks for responding. Unfortunately I do have those backticks in they just don't always translate considering I haven't used the markdown cheatsheet yet. Let me try this:
let [red, green, blue] = [0];
let randomRGB;
let html = '';
const main = document.querySelector('main');
function getNumber() {
return Math.floor(Math.random() * 256);
}
red = getNumber();
green = getNumber();
blue = getNumber();
console.log(red, green, blue);
for (i = 0; i <= 10; i++) {
let randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
console.log(randomRGB);
html += `<div style=background-color: ${randomRGB}">${i}</div>`;
}
main.innerHTML = html;
That's still giving me the same white backgrounds.
Edit: as a side note, can you tell me what the quotations after ${randomRGB} (in the for loop) are there for? They were in the default workspace code and I'm a little confused as to why there is only one set.
Thanks again for all your help.
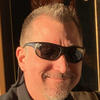
Peter Vann
36,425 PointsHi Casey
One issue right off the bat is there is a double-quote missing in this line:
html += `<div style=background-color: ${randomRGB}">${i}</div>`;
(After style= )
It should be:
html += `<div style="background-color: ${randomRGB}">${i}</div>`;
Try something more like this:
//let [red, green, blue] = [0]; // Not necessary from what I can tell!?!
let randomRGB;
let html = '';
const main = document.querySelector('main');
function getNumber() {
return Math.floor(Math.random() * 256);
}
for (i = 0; i <= 10; i++) {
let red = getNumber();
let green = getNumber();
let blue = getNumber();
console.log(red, green, blue);
let randomRGB = `rgb(${red}, ${green}, ${blue})`;
console.log(randomRGB);
html += `<div style="background-color: ${randomRGB}">${i}</div>`;
}
main.innerHTML = html;
I hope that helps.
Stay safe and happy coding!
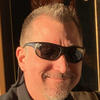
Peter Vann
36,425 PointsHi Casey!
You are still missing the first double-quote in this line:
html += `<div style=background-color: ${randomRGB}">${i}</div>`;
^ ^
(Here) (To match this one)
(Before the word background)
But I have also experienced this:
One thing I have discovered, which is really weird and baffling, is every once in a while, I will type code that I know is 100% correct, but JUST WON'T PASS!?! So let's say the challenge wants a variable to equal the text "I am a great programmer!" And if I hand type the text myself, it fails, but if copy and paste the text from the challenge text itself, then it passes EVEN THOUGH IT IS VERBATIM THE SAME!?! It is a workaround I discovered just by trial-and-error. I have NO IDEAL why it would make a difference, but somehow it does!?!
One tool I use which helps me spot typos (and any differences in two seemingly identical pieces of code) is this:
I hope that helps.
Stay safe and happy coding!
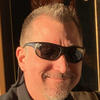
Peter Vann
36,425 PointsCasey,
You are certainly welcome!
It feels nice to help out anyone struggling with the challenges.
Coming up through the ranks, I have certainly had my share of challenges that I just could not make pass and have nearly literally pulled my hair out trying to figure out the issue (it often not being even improper syntax)!?!
It really sucks when you are stuck on a technical issue or a lame typo oversite(or even it seeming like maybe the video didn't cover the topic thoroughly enough)!?!
So any wisdom, tips, and tricks I can pass along, I surely do.
I'm sure you'll pay it forward, too, when you can!
Have a great (rest of the) weekend!
-Pete
PS: You may find this fun - my take on the classic "Simon Game*" coding challenge (LOL):

Casey Beaver
5,619 PointsThank you so much Peter!
That " made it work! But more importantly, I appreciate your patience and the code you provided because it made me think in a different way. I really can't stress that enough.
This is such a great community to learn. It's unlike any I've ever been a part of.
Cheers!
Casey Beaver
5,619 PointsCasey Beaver
5,619 PointsHi Peter,
So your code worked when I pasted it into both the link you provided AND the workspace editor (I only copied what's between the <script> tags for the workspace editor while copying all of it for link.
What's really weird is when I go back to what I had and rewrite it exactly as you did, I get no backgrounds. Unless I'm crazy I have it character for character. Here's what I've got that isn't working (while yours does):
Also, here is my html