Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial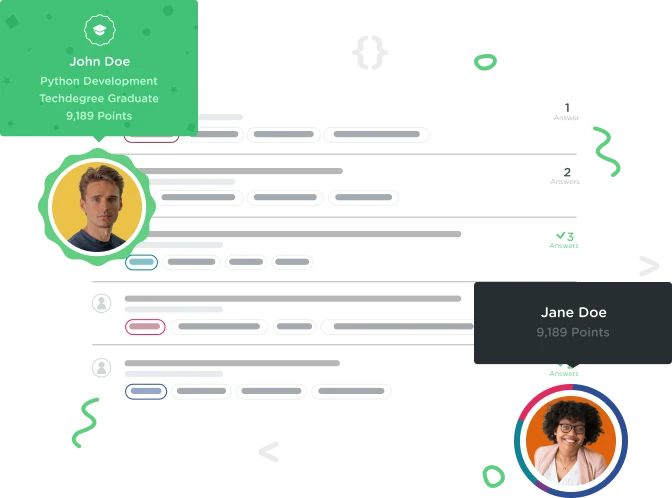

Aditya verma
Courses Plus Student 664 PointsCant get this one right
help me where I am wrong
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors(){
Set<String> allAuthors = new TreeSet<String>();
for( Blog bp : posts ){
allAuthors.add(bp.getPosts());
}
return allAuthors;
}
}
4 Answers

Hassaan Ahmad
1,979 PointsHey Aditya, I see a couple of mistakes in your getAllAuthors() method.
- To get the posts in your for loop, you need to call the getPosts() method defined above. +The variable 'bp' that you used has to be of the type BlogPost for the loop to work as it'll run through a list of Blog Posts. Makes sense? Hope that helps

Aditya verma
Courses Plus Student 664 PointsThat fine , but I never created an object in the blogPost class. If you see in the tutorial they have created objects and then called.

Aditya verma
Courses Plus Student 664 Pointspublic Set<String> getAllAuthors(){ Set<String> allAuthors = new TreeSet<String>(); for( BlogPost bp : getPosts() ){ allAuthors.add(bp.getPosts()); } return allAuthors; }
can yo help me with this part of code

Hassaan Ahmad
1,979 PointsYou don't have to create a new instance of BlogPost object as the getPosts() method returns a list of BlogPost objects. As they are already of type BlogPost, you can use on them the methods offered by the BlogPost class.
Coming to your second question, as I see, getAllAuthors() method returns the Set of all authors.
- The first line instantiates a new Set which is to be filled by the authors.
- The statement of for loop goes like for each bp of type BlogPost in the List returned by getPosts(), get the author and add it to the allAuthors Set.
- After the loop ends, return the Set of allAuthors.
In your code, I see that in the for loop, you are adding bp.getPosts() instead of bp.getAuthor() to the Set. Remember that you are supposed to add the authors in the Set.