Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial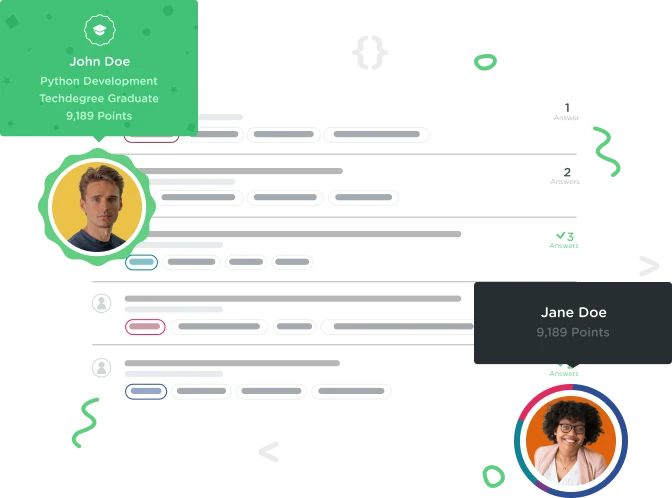
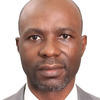
Babatunde Obidare
Full Stack JavaScript Techdegree Student 4,925 PointsCan't get this to compile
Return the average length of the tongues of the frogs in the array. Use a for loop as part of your solution
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int TongueLength[] = tongueLength[frogs.Length];
double totalTongueLength = 0;
for (int i = 0; i < frogs.Length; i++)
{
totalTongueLength = totalTongueLength + tongueLength[i];
}
return totalTongueLength / frogs.Length;
}
}
}
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int TongueLength[] = tongueLength[frogs.Length];
double totalTongueLength = 0;
for (int i = 0; i < frogs.Length; i++)
{
totalTongueLength = totalTongueLength + tongueLength[i];
}
return totalTongueLength / frogs.Length;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers

andren
28,558 PointsThere are two errors in your code, the first is this entire line:
int TongueLength[] = tongueLength[frogs.Length];
First of all there is no variable in the FrogStats class called tongueLength, secondly by using those brackets on the variable you are saying that you want to extract one item from that array, and you cannot set an array to a single item.
I'm going to assume based on looking at the rest of the code, that you intended this line to create an array filled with the Tongue lengths of all of the frog objects.
The second problem is this line:
totalTongueLength = totalTongueLength + tongueLength[i];
As mentioned above tongueLength is not a variable defined in this class, though I assume that this is a typo and you meant to refer to the variable you declared at the top.
Your code can be fixed by making two small changes, I'll first post the corrected code then explain how it works:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double totalTongueLength = 0;
for (int i = 0; i < frogs.Length; i++)
{
totalTongueLength = totalTongueLength + frogs[i].TongueLength;
}
return totalTongueLength / frogs.Length;
}
}
}
The first change is to remove your TongueLength variable, because it was syntactically incorrect and not actually needed to complete the challenge, and the second is to change tongueLength[i] to frogs[i].TongueLength.
The frog object has a property called TongueLength as can be seen by looking at the frogs.cs file, this property can be used to get the length of the frog's tongue, using that in place of the variable you thought held all of the tongue lengths ends up making the rest of your code work the way you intended it to.
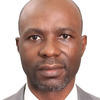
Babatunde Obidare
Full Stack JavaScript Techdegree Student 4,925 PointsThanks Andren! The explanation is well understood.