Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial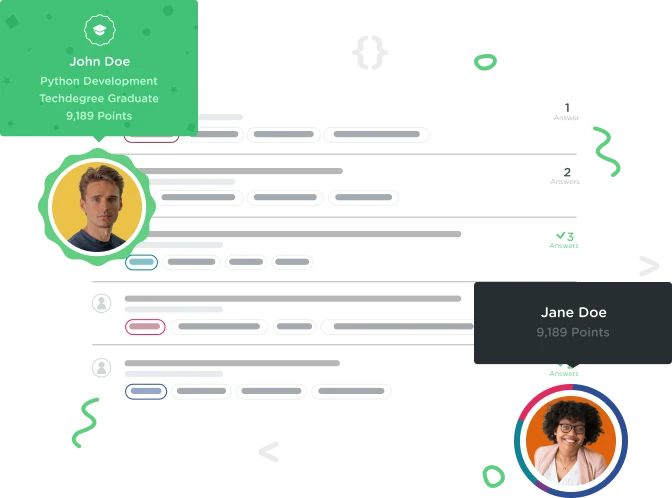

Tiago Ramos
2,380 PointsCan't I remove the vowels one by one?
I know this is not the most elegant way, but it should work right?
Can somebody help me with the logic?
def disemvowel(word):
word.remove("a")
word.remove("e")
word.remove("i")
word.remove("o")
word.remove("u")
word.remove("A")
word.remove("E")
word.remove("I")
word.remove("O")
word.remove("U")
return word
1 Answer
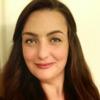
Jennifer Nordell
Treehouse TeacherHi there! No, unfortunately this won't work. You might try your code in a workspace and see the results. But the bottom line is that you'll get an error. We're sending in a string and strings are immutable. The remove
method is specifically for lists. Also, even if remove did work here, it wouldn't remove every instance of a letter. For example, what if you sent in "Balloon"? You need to account for repeating vowels as well.
Hope this helps!
edited for additional hints
Here are some hints:
- Try making a new empty string
- Try iterating through the string sent in
- For every letter that isn't one of those letters add it to your new string
- When you're done iterating through the string sent in, return the new string you built
Tiago Ramos
2,380 PointsTiago Ramos
2,380 PointsHi Jennifer thanks for those hints. I've tried something completly new, with some of your tips, but I don't know how iterate.
def disemvowel(word): word_list = word.list() vowels = ["a", "e", "i", "o", "u"]
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi again, Tiago! Yes, I can see you've worked on this and that's great! And you are, in fact, iterating. Let me show you how I did it and I'll walk you through it:
So we start by defining a function that will receive a word as a string. That was already done for us by Treehouse. Next, I set up a vowels string to contain all the letters we don't want in our returned string. Then I set up a string to hold the string we're going to return back to Treehouse.
Now I go through the string they sent in by saying for every letter in this word, take a look at that letter. If the letter is contained in the
vowels
string, don't do anything at all. Just move on to the next letter, which is what thecontinue
statement does. However, if it wasn't a vowel, please add it to mynew_word
. When we're done looking at all the letters inword
what we'll have is anew_word
that has only had the consonants appended to it. Please return thenew_word
to the piece of code that asked for it.Hope this helps clarify things!