Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial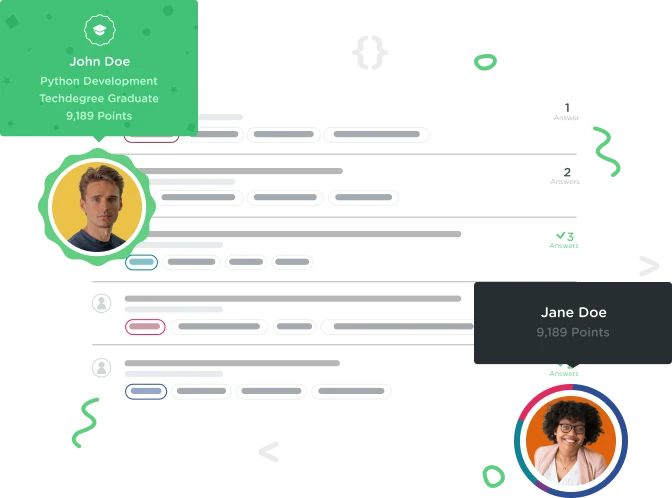

Hamzah Iqbal
Full Stack JavaScript Techdegree Student 11,145 PointsCan't i use this approach regarding the module export?
const express = require('express');
const router = express.Router();
/*
* Helpers for Various Tasks
*/
// Helper function to reverse a string
const reverseString = (string) => [...string].reverse().join('');
// Helper function to shorten a string to fifty characters
const shortenString = (string) => {
return string.length > 50 ? string.substring(0, 50) + "..." : string;
}
module.exports = reverseString;
module.exports = shortenString;
Then access it in the app.js as:
helper.reverseString helper.shortenString It is just named functions, so it should be able to do so, right?
2 Answers
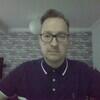
Brian Wright
6,770 PointsThink of module.exports as an standard empty js object {}.
If you run module.exports = reverseString, module.exports now becomes the function. if you then run module.exports = shortenString;, you are reassigning module.exports as a new function.
What you need to do is add the functions as methods of the module.exports object with
module.exports.reverseString = reverseString and module.exports.shortenString = shortenString or simpler as
module.exports = { reverseString , shortenString }. module.exports is now an object with two methods.
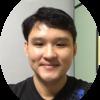
Boon Kiat Seah
66,664 Pointsmodule.exports = reverseString; module.exports = shortenString;
If you will like to use module.exports with two different functions, the way you have done will not work. It best to write it this way
module.exports = {
reverseString,
shortenString
};
Next, to import into the file, the code should look something like this
const helperMethods = require('./helperMethods');
helperMethods.reverseString("Some String");
Hope this helps