Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial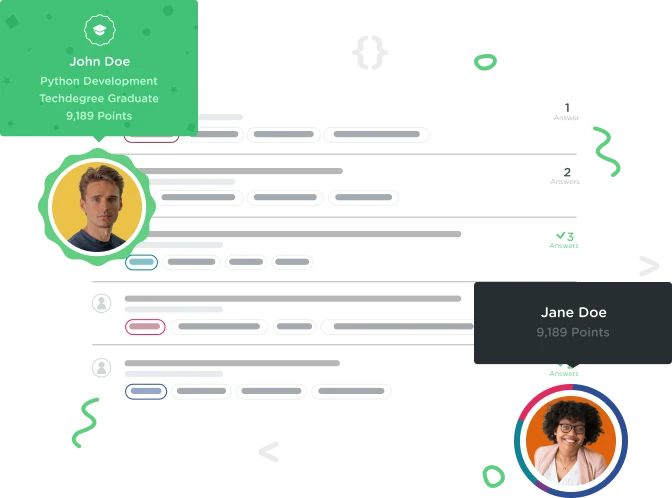

Jasper Peijer
45,009 PointsCan't load static files
I've came across another issue when trying to load a static file. If I try to do that I get the following warning:
WARNING: An illegal reflective access operation has occurred
WARNING: Illegal reflective access by com.github.jknack.handlebars.context.MemberValueResolver (file:/C:/Users/myname/.gradle/caches/modules-2/files-2.1/com.github.jknack/handlebars/4.0.6/ccf00179b6648523e5c64b9b5fb783d89e42401b/handlebars-4.0.6.jar) to method java.util.Collections$EmptyMap.isEmpty()
WARNING: Please consider reporting this to the maintainers of com.github.jknack.handlebars.context.MemberValueResolver
WARNING: Use --illegal-access=warn to enable warnings of further illegal reflective access operations
WARNING: All illegal access operations will be denied in a future release
[qtp1458483009-23] INFO spark.http.matching.MatcherFilter - The requested route [/favicon.ico] has not been mapped in Spark for Accept: [image/webp,image/apng,image/*,*/*;q=0.8]
It is causing my webpage not to load.
I've tried changing my Java version to 8 instead of 11. But no changes.
base.hbs:
{{#block "header"}}
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{{#block "title"}}Welcome to Course Ideas{{/block}}</title>
<link rel="stylesheet" href="/css/main.css">
</head>
<body>
{{/block}}
{{#block "content"}}{{/block}}
{{#block "footer"}}
</body>
</html>
{{/block}}
index.hbs:
{{#partial "content"}}
{{#if username }}
<h1>Welcome {{ username }}</h1>
{{ else }}
<h1>Welcome Students!</h1>
<form action="/sign-in" method="post">
<input type="text" placeholder="What's your username?" name="username" />
<button>Sign In</button>
</form>
{{/if}}
{{/partial}}
{{> base.hbs}}
Main.java
package com.jasper.courses;
import com.jasper.courses.models.CourseIdeaDAO;
import com.jasper.courses.models.SimpleCourseIdeaDAO;
import spark.ModelAndView;
import spark.template.handlebars.HandlebarsTemplateEngine;
import java.util.HashMap;
import java.util.Map;
import static spark.Spark.*;
public class Main {
public static void main(String[] args) {
staticFiles.location("/public");
SimpleCourseIdeaDAO dao = new SimpleCourseIdeaDAO();
get("/", (req, res) -> {
Map<String, String> model = new HashMap<String, String>();
model.put("username", req.cookie("username"));
return new ModelAndView(model, "index.hbs");
}, new HandlebarsTemplateEngine());
post("/sign-in", (req, res) -> {
Map<String, String> model = new HashMap<String, String>();
res.cookie("username", req.queryParams("username"));
model.put("username", req.queryParams("username"));
return new ModelAndView(model, "sign-in.hbs");
}, new HandlebarsTemplateEngine());
}
}
I've tried both:
staticFileLocation("/public");
and
staticFiles.location("/public");

Jasper Peijer
45,009 PointsIt doesn't cause a single error, only the warnings I put in the post above. I have tried staticFileLocation("/public");
but decided to try the other way because it didn't work and the Sparks documentation listed this. The folders are in the correct place and commenting out the link tag causes the page to load properly.

saykin
9,835 PointsSo when you don't map your css static file, the page loads? What's the code in your css file?

Jasper Peijer
45,009 Pointsbody {
background-color: '#1f1f1f';
color: white;
}
I'm really stupid, I've just realized that I shouldn't put color codes inbetween quotes in native css. I've been working a lot with Flutter and React Native lately where it is required. Fixed the CSS and it's working now, thanks anyways!
2 Answers

Jasper Peijer
45,009 PointsChanges in main.css:
body {
background-color: '#1f1f1f';
color: white;
}
to:
body {
background-color: #1f1f1f;
color: white;
}
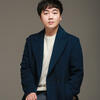
Jade Kang
Courses Plus Student 8,424 PointsI just had the same issue and noticed that the file path should not be /public because the resources are in our src directory so you should name it public.css for the path. It worked for me. Try to change to this:
staticFileLocation("/public.css");
saykin
9,835 Pointssaykin
9,835 PointsI honestly can't see the issue with this code, except for the staticFiles.location. In my code from this course I use staticFileLocation("/public");
I'd like to add that I get these same warnings, but my webpage does load.
Are your static files in \src\main\resources\public folder? What error message do you get?