Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial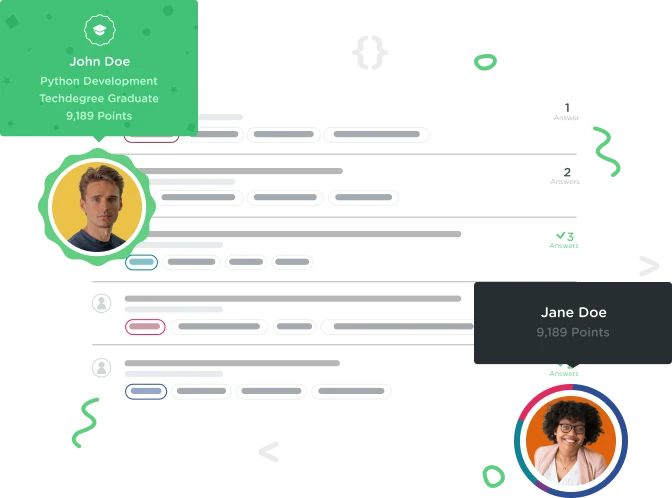

Melissa Bonnici
2,190 PointsCan't loop through multiple dictionaries
So I am trying to get the proper thing to return on this challenge, which is a list of strings. I can get the code to work for one dictionary, but I have tried a variety of different things to get it to work on a list of multiple dictionaries and nothing is working. Could someone please let me know what is necessary to get multiple dictionaries in a list to work and return the proper statement??
Thank you so much in advance!!
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
def string_factory(name=None, food=None):
list_of_strings = []
if name and food:
list_of_strings.append(("Hi, I'm {} and I love to eat {}!".format(name, food)))
print(list_of_strings)
string_factory(**{"name": "Michelangelo", "food": "PIZZA"})
#string_factory(values)
1 Answer
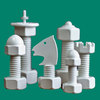
Steven Parker
229,785 PointsYou need a loop.
If you called the incoming argument "dictlist", you might write something like: "for onedict in dictlist:
".
Some other hints that may help:
- don't replicate the template, use they one provided (by name)
- try the advice in the instructions about "using ** for each dictionary in the list"
- just define the function, don' call it (the challenge does it itself)
- remember to return the final string list
- you won't need to print anything
Melissa Bonnici
2,190 PointsMelissa Bonnici
2,190 Points"using ** for each dictionary in the list"
I am confused as to how to both keep the incoming argument a dictlist and do the above. I attached my code below. So if the incoming argument is a list of dictionaries, how can I do **kwargs as the incoming argument like I normally would?
Thank you!
def string_factory(dictlist): list_of_strings = [] for one_dict in dictlist: for kwargs in one_dict: name = None food = None if name and food: list_of_strings.append(("Hi, I'm {} and I love to eat {}!".format(name, food))) return list_of_strings
Steven Parker
229,785 PointsSteven Parker
229,785 PointsTo make posted code readable, always use the formatting instructions found in the Markdown Cheatsheet below the "Add an Answer" area.
You don't need 2 loops. Combining my first two hints, you can replace the second loop with just this:
list_of_strings.append(template.format(**one_dict))