Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial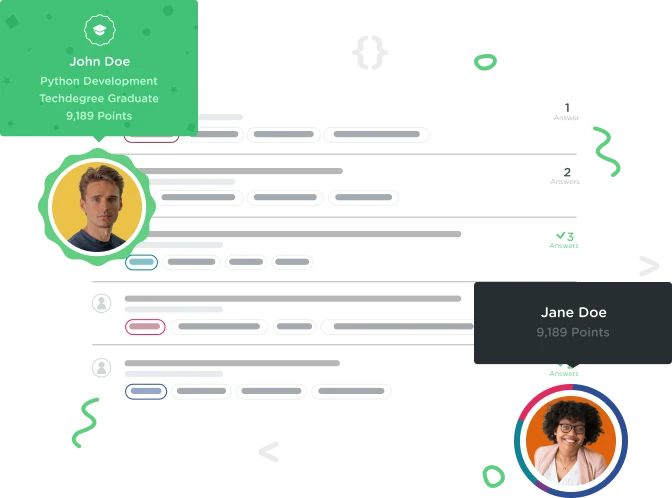

Louis Baerentzen
2,892 PointsCant make the throw illegal argument exception to work
I am currently on the penultimate stage of the go-kart challenge and am unsure on how to express the Illegal argument exception so that the battery charge and lap count do not conflict with each other.
Any help on this is much appreciated.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int laps = lapsDriven ++ laps;
int charge = barCount -- laps;
if barCount > 0; {
throw new IlllegalArgumentException("Charge battery");
}
}
}
1 Answer
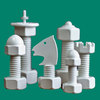
Steven Parker
231,269 PointsHere's a few hints:
- leave the original code lines as they were to handle cases where there is no error
- do the test/throw first in the method, before anything is modified
- the test should compare the argument (request) with the available energy
- there should not be a semicolon between an "if" expression and the brace starting the code body
Louis Baerentzen
2,892 PointsLouis Baerentzen
2,892 PointsHello Steven,
Thank you for your hints on the challenge I've posted about.
Im really not finding it easy to grasp the suggestions to the challenge that you have sent me. The concept of objects and how everything is in reference to each other Im struggling with towards understanding what to change and where.
Do you have any advice on how to break the concepts down, Im just not getting it?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe code inside the method is just dealing with arguments and variables and won't need anything specific to objects. Show your new code after you've applied the hints if you still have trouble.
Louis Baerentzen
2,892 PointsLouis Baerentzen
2,892 PointsI have had success with this answer to that challenge, thank you again for the help Steven.
Louis Baerentzen
2,892 PointsLouis Baerentzen
2,892 PointsI had another quick query based on the commenting I leave per line.
Do you recommend this as a way to help breakdown what is happening when learning java at this stage?
Im finding it helpful to breakdown what the code is doing in a commented sentence aside the lines of actual code.
The concept of OOP is quite new to me and my background in code is only with HTML and CSS which are of course mark up languages.
Any thoughts you have on this and how to wrap ones head around the flexibility of java with the way its oriented via objects would be priceless.
Kind Regards Louis
Steven Parker
231,269 PointsSteven Parker
231,269 PointsBreaking the steps down is often helpful to understanding, I still do it occasionally when looking at some complex code.
Glad I could help. You can mark the question solved by choosing a "best answer".
And happy coding!