Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial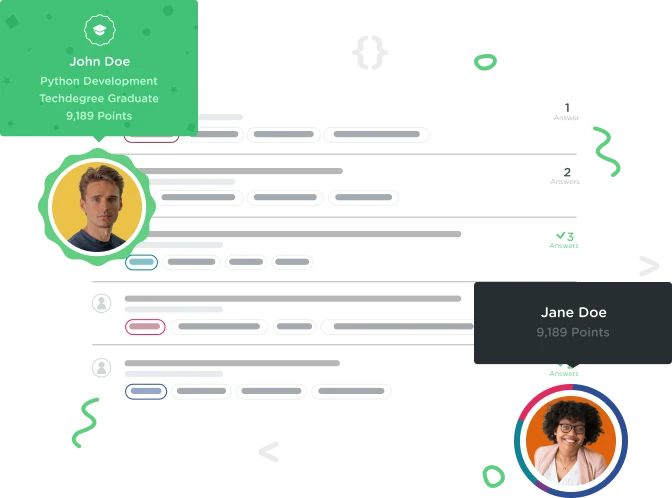

Andy Hughes
10,049 PointsCan't output the right answer from my comparison test script
After watching the videos, I have tried to create what I think is a simple test script. The script is aimed at checking competition entries and then outputting the winner. The initial variables, I have just set to simulate entries with one being correct.
The next part should check to see if there is only one winner. It then moves on to check which contestant is the winner. This is where it seems to be going wrong for me. The result should be that Contestant Two is the winner, but instead it tells me Contestant One has won.
I can't move on until I know what I'm doing wrong and why. If I can't understand it, I can't carry on. Looking for some help, thanks. the code is below.
<?php
$contestant_one = false;
$contestant_two = true;
if ($contestant_one == TRUE && $contestant_two == TRUE) {
print("Only one winner!");
}
else {
$contestant_one = "Contestant One";
$contestant_two = "Contestant Two";
if ($contestant_one == TRUE){
echo 'Congratulations '. $contestant_one .' you are the winner';
}
else
{
echo 'Congratulations '. $contestant_two .' you are the winner';
}
}
?>
2 Answers

Craig Watson
27,930 PointsHi Andy :)
It seems because you are declaring the same variable name even though it is within the function as soon as you change that name you can get the results you would expect.
This could be different if you set the above code up as a php function and declared the variables within the function scope.
I hope you don't mind I played around with it a little to leave no blank screen if both were false.
<?php
$contestant_one = false;
$contestant_two = false;
$contestant_one_name = "Contestant One";
$contestant_two_name = "Contestant Two";
$only_one_winner = "There can only be one winner!";
$no_winners_today = "Bummer nobody won today, better luck next time!";
// Check both contestants are not winners
if ( $contestant_one === TRUE && $contestant_two === TRUE ) {
echo $only_one_winner;
// Check if nobody won at all
} else if ( $contestant_one === FALSE && $contestant_two === FALSE ) {
echo $no_winners_today;
} else {
if ( $contestant_one === TRUE ) {
echo 'Congratulations '. $contestant_one_name .' you are the winner';
} else if ( $contestant_two === TRUE ) {
echo 'Congratulations '. $contestant_two_name .' you are the winner';
}
}
?>
I think a great step further forward here would be to switch this into using an array for the contestants :)
Hope this helps
Craig

Maximillian Fox
Courses Plus Student 9,236 PointsIt seems you are declaring your contestant variables again later on down in the code, and changing their values, which will cause your if true part to always return contestant one.
<?php
$contestant_one = false;
$contestant_two = true;
if ($contestant_one == TRUE && $contestant_two == TRUE) {
print("Only one winner!");
} else {
//The variables have been re-declared, so if($contestant_one == TRUE) will return true because the variable has been defined.
//$contestant_one = "Contestant One";
//$contestant_two = "Contestant Two";
//Change them to the following:
$contentant_one_string = "Contestant one";
$contestant_two_string = "Contestant two";
// This if statement
if ($contestant_one == TRUE){
echo 'Congratulations '. $contestant_one_string .' you are the winner';
} else {
echo 'Congratulations '. $contestant_two_string .' you are the winner';
}
}
?>
Just changing the variable names will solve this for you.
As a note
<?php
if($variable == true)
?>
will ALWAYS return true if $variable is set, has a nonzero or non-boolean-false value, and not null. To tighten up your code even more, you should use
<?php
if($variable === true)
?>
as this will also ensure $variable is a boolean value set to true.

Andy Hughes
10,049 PointsI think I get this, so I need to create a different variable so it doesn't just redeclare the first variable yes? Even with this as Craig mentioned, I get a blank page if results are both false. :)

Maximillian Fox
Courses Plus Student 9,236 PointsCorrect - you will need to create new variables with different names.
Or, if you are familiar with arrays, you could look into using those which will allow you to have more than 2 contestants, so you could accept as many or as few as you wanted to in your script.
Happy coding! :)
Andy Hughes
10,049 PointsAndy Hughes
10,049 PointsI think I get this now Craig, although it will be some time before it is second nature. The videos are great, but I just need to be able to know I myself can practically put the steps into actual in a real-world type scenario. Hence the script above.
Thanks for your help.
Craig Watson
27,930 PointsCraig Watson
27,930 PointsHi Andy,
I learn in the same way to be honest I can watch videos all day and nothing really goes in I have to follow along and make a couple of fake projects for myself to really test me out and push what I have learned.
You are certainly doing the right thing I'd say by practicing a little and if you get stuck at a particular point, as you have done here hit up the community and there is always someone willing to give you a hand!
Are you following the PHP Developers Track ?
Craig