Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial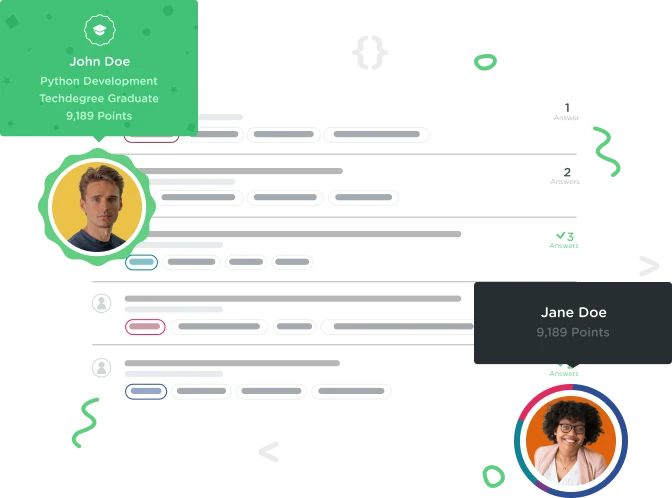

David Prince
5,799 PointsCan't pass the challenge. When I modify code from Treehouse's github it cannot find symbol for .getCriteriaBuilder.
I've spent a lot of time on this, looked at other's questions about it. Tried having it return null. I'm pretty lost.
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
}
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Hibernate;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao {
@Autowired
private SessionFactory sessionFactory;
@Override
@SuppressWarnings("unchecked")
public List<Contact> findAll() {
// Open a session
Session session = sessionFactory.openSession();
// DEPRECATED as of Hibernate 5.2.0
List<Contact> contactss = session.createCriteria(Contact.class).list();
// Create CriteriaBuilder
CriteriaBuilder builder = session.getCriteriaBuilder();
// Create CriteriaQuery
CriteriaQuery<Contact> criteria = builder.createQuery(Contact.class);
// Specify criteria root
criteria.from(Contact.class);
// Execute query
List<Contact> contacts = session.createQuery(criteria).getResultList();
// Close session
session.close();
return contacts;
}
}
1 Answer
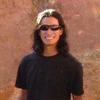
Daniel Vargas
29,184 PointsHi
I struggled with this challenge as well, it turns out to be a little simpler than you think
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
}
And in the ContactDaoImpl you just had to annotate the class and implement the method, but you didn't need to add a behavior:
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao {
@Override
public List<Contact> findAll(){
return null;
}
}