Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial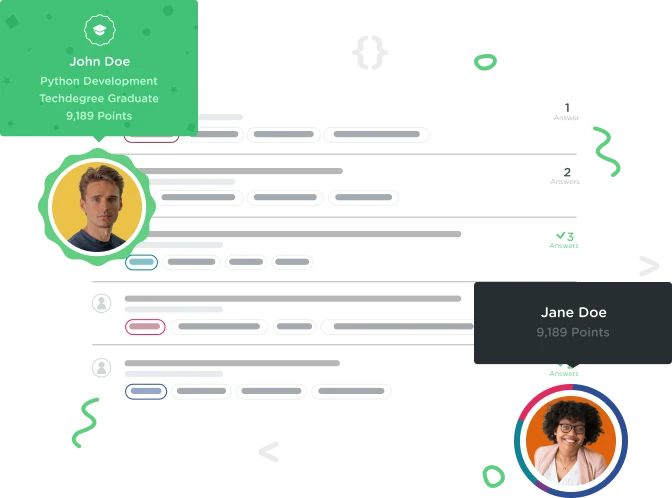

Lingjian Kong
6,330 PointsCan't pass the set challenge.
Hi. Can someone tell me what's wrong with my code?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.*;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import jave.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> allAuthors = new TreeSet<String>();
for(BlogPost each: mPosts){
allAuthors.addAll(each.getAuthor());
}
return allAuthors;
}
}
1 Answer

andren
28,558 PointsYour code is pretty close to correct there are just two issues:
You have a typo in the last import statement. You misspelled java as jave.
addAll
is a method used to add the contents of a collection to a set, not for adding individual items like you are doing. The method for adding individual items is simplyadd
.
If you fix those two issues then your code should work.
Though It's also worth mentioning that your import statements are a bit messy. You first import java.utils.*
which imports everything from that namespace, and then you also import the individual things you need which is redundant. You should stick to either importing everything or importing the specific things you need.
In this case I'd recommend against importing everything since you are only using a couple of classes from java.utils
. Removing your first import statement won't affect your code at all.