Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial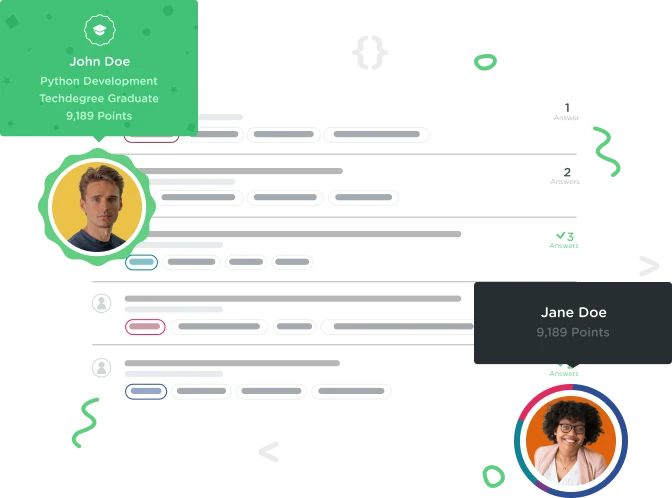

Joe Tran
4,768 PointsCan't pass through the compile error
when i try to check my work, it keeps saying that a compile error happens at != in line 8, does anyone know the error?
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private String normalizeDiscountCode(String discountCode) {
for (char letter : discountCode.toCharArray()) {
if (!Character.isLetter(letter) && (letter != "$")) {
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
try {
this.discountCode = normalizeDiscountCode(discountCode);
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage());
}
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers
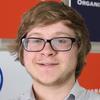
Cam Richardson
Front End Web Development Treehouse Moderator 16,895 PointsThe error is this:
./Order.java:8: error: incomparable types: char and String
if (!Character.isLetter(letter) && (letter != "$")) {
Which means that you are trying to compare letter (a char) and "$" (a string). This is because in Java (and C# I believe) you must denote a character literal with single quotes like this:
char letter = 'A';
If you don't do this, the compiler will think you're meaning to define a String literal even if the string is a single character. Give it a try and let me know if that helps! :)

Joe Tran
4,768 PointsOops, i find out the reason.
It is because the question has not asked me to catch the exception....
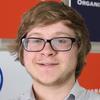
Cam Richardson
Front End Web Development Treehouse Moderator 16,895 PointsGlad to hear you got it working!
Joe Tran
4,768 PointsJoe Tran
4,768 PointsThx Richarson!
I have changed it to single quote and it compiled successfully!
However, I bumped into a new error:
Bummer: Hmmm...I ran order.applyDiscountCode("h1!") and I expected it to throw an IllegalArgumentException, but it didn't
Why cant the code throw the error in this h1! example.... any help?