Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial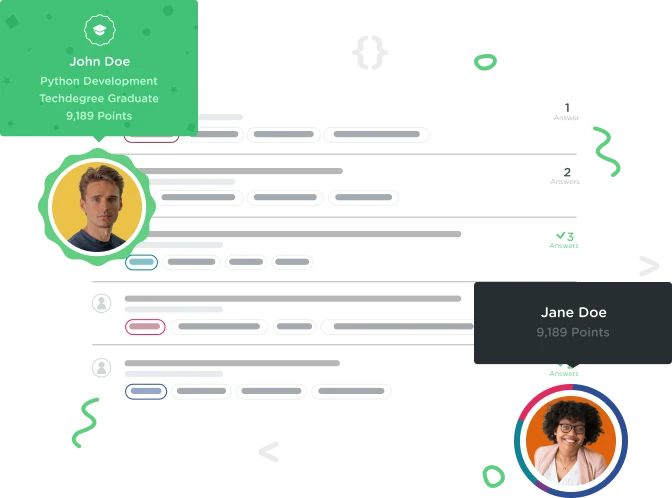

Diego Petrucci
6,033 PointsCan't properly nest two swift functions
I'm doing the second part of the task.
I need to assign the result of the nested function to a constant named difference. Xcode says it's okay, the treehouse editor says it's not.
/**
For this code challenge, letβs define a math operation as a function that
carries out some work on two integers and returns an integer as well. An
example is the function below, `differenceBetweenNumbers`, which takes two
integers and calculates the difference between the numbers. After calculating,
it returns the difference.
*/
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation(aMathOperation: ((Int, Int) -> Int), firstInt: Int, secondInt: Int) -> Int {
return (aMathOperation(firstInt, secondInt))
}
let difference = mathOperation(differenceBetweenNumbers, 6, 2)
2 Answers
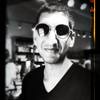
Taha Abbasi
12,865 PointsHello,
After reading the documentation I got it to work like below:
/**
For this code challenge, letβs define a math operation as a function that
carries out some work on two integers and returns an integer as well. An
example is the function below, `differenceBetweenNumbers`, which takes two
integers and calculates the difference between the numbers. After calculating,
it returns the difference.
*/
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation(mathFunction: (Int, Int) -> Int, a: Int, b: Int) -> Int {
return mathFunction(a, b)
}
let difference = mathOperation(differenceBetweenNumbers, 10, 5)
In the mathOperation function make sure the first parameter is a function and you are returning the result of that function.
func mathOperation(mathFunction: (Int, Int) -> Int, a: Int, b: Int) -> Int {
return mathFunction(a, b)
}
You can find more details in the Function Types as Parameter Types section of the docs here:

Jason Harrison
Courses Plus Student 2,868 PointsThanks, Taha. Man, I swear that's what I tried first. Either way, it works. Moving on!

Bruno Bandeira de Azevedo
25,374 PointsIn my case it was the parenthesis also. There is a difference for the challenge checker between:
func mathOperation(mathFunction: (Int, Int) -> Int, a: Int, b: Int)->(Int){}
and
func mathOperation(mathFunction: (Int, Int) -> Int, a: Int, b: Int)->Int{}
Weird but worked for me =P.
Jason Harrison
Courses Plus Student 2,868 PointsJason Harrison
Courses Plus Student 2,868 PointsI'm having the same trouble. I've been having some inconsistency with the Preview window, as well. It is often out of sync with my code (showing errors on line numbers already corrected, for example). In this case, it's entirely blank so I don't even have a suggestion of what might be wrong.
On another note, thank you for providing your code snippet! I was stuck on the first step of the exercise because I didn't wrap my function type in parens like you did.
Hopefully there's a solution to this problem that is something other than "the compiler isn't working". I'll share one if I find it.