Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial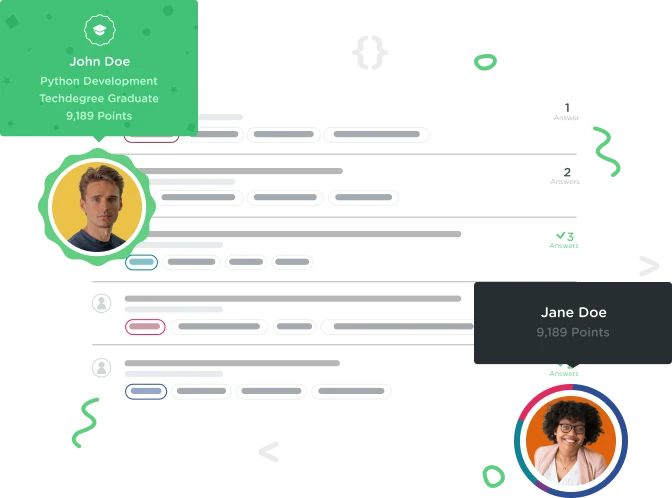
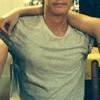
William Hartvedt Skogstad
5,857 PointsCan't really grasp the concept of Tuples..
Need a little walkthrough on this Tuple challenge :)
func greeting(person: String) -> String {
let language = "English"
let greeting = "Hello \(person)"
return greeting
}
4 Answers
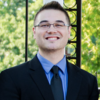
ianhan3
4,263 PointsThe greeting function above basically just returns a String value. You want to return two values, each of type string, based on the values given in the function.
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
So you want to tell the function to return two strings in the function declaration then do the same in the return statement as well. Does that make sense?
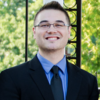
ianhan3
4,263 PointsIn the second exercise, they ask you to: "Create a variable named result and assign it the tuple returned from function greeting. (Note: pass the string "Tom" to the greeting function.)"
Super simple, just follow exactly what they say.
- "Create a variable named result"
var result =
- Assign it to the tuple returned from the function "greeting"
var result = greeting()
- Pass it the string "Tom" into the greeting function
var result = greeting("Tom")
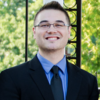
ianhan3
4,263 PointsThird is just dot notation to access the value.
"Using the println statement, print out the value of the language element from the result tuple."
println(result.language)
Note: In Swift 2.0 println() just becomes print() but for the sake of the exercise it's still Swift 1.X
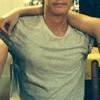
William Hartvedt Skogstad
5,857 PointsThanks a lot for such a detailed walkthrough! It really helped to get an understanding of how Tuples work :)
William Hartvedt Skogstad
5,857 PointsWilliam Hartvedt Skogstad
5,857 PointsHere is the Challenge:
Currently our greeting function only returns a single value. Modify it to return both the greeting and the language as a tuple. Make sure to name each item in the tuple: greeting and language. We will print them out in the next task.