Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial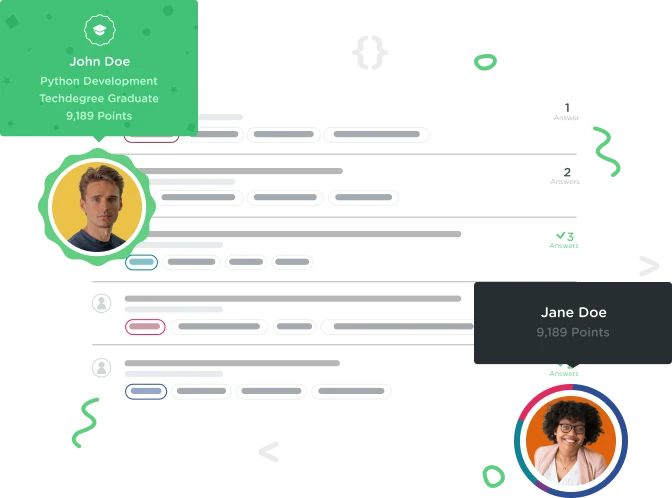

changsoon jang
Courses Plus Student 1,731 PointsCan't resolve Challenge Task
Assume you are creating a Weather app and are provided the temperature in Celsius. We need to create a read-only computed property called Fahrenheit which will return a computed value. (Note: Fahrenheit = (Celsius * 1.8) + 32)
and given some code:
class Temperature {
var celsius: Float = 0.0
}
class Temperature {
var celsius: Float = 0.0
}

changsoon jang
Courses Plus Student 1,731 PointsOh, I made it. I was confused with uppercasing the first letter,because in the task it gives 'F'ahrenheit and 'C'e'sius.
class Temperature { var celsius: Float = 0.0 var fahrenheit: Float { return celsius * 1.8 + 32 } }
3 Answers
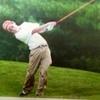
kjvswift93
13,515 Pointsclass Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
return (celsius * 1.8) + 32
}
}
Computed properties do not actually store a value in memory, so instead of assigning a value to the fahrenheit computed property we create here, it is used to calculate and return the respective fahrenheit value for temperature. Read only computed properties, like this one here, has a getter but no setter, and always returns a value that cannot be set to a different value. (Hence read-only) However, it still needs to be declared using the keyword var, despite it's seemingly constant-like behavior, because it's value is still not fixed.
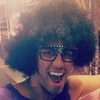
David Maybach
2,092 PointsA more complete calculation is here.
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
return celsius * 1.8 + 32
}
init (celsius: Float) {
self.celsius = celsius
}
}
let weather = Temperature (celsius: 1)
weather.fahrenheit
This answer above will calculate the conversion. Hope this helps.
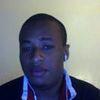
Abdoulaye Diallo
15,863 Pointsclass Temperature { var celsius: Float = 0.0 var fahrenheit: Float { return (celsius * 1.8) + 32 } }
P.S. In the Question: C is capitalized and the code has a lower class C.
Justin Black
24,793 PointsJustin Black
24,793 PointsThey give you the formula, and the video right before it clearly shows how to create a computed property. Review the video, and look at this question again.