Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial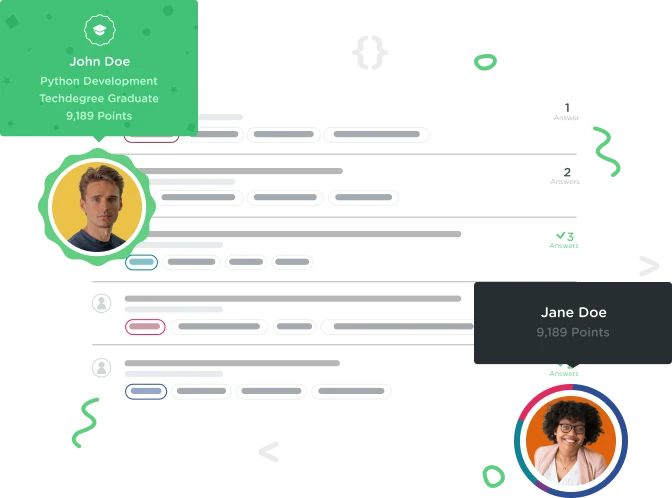

Akshay Rathee
1,205 Pointscan't resolve symbol "AlertDialogFragment"
hi guys, i keep getting this error can't resolve symbol "AlertDialogFragment". can you help me? Thanks in advance
5 Answers
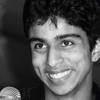
Dhruv Gupta
4,320 PointsIf you didn't go to one of your folders, probably UI.
Create a new class and paste the following code:
public class AlertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context)
.setTitle(context.getString(R.string.error_title))
.setMessage(context.getString(R.string.error_message))
.setPositiveButton(context.getString(R.string.error_ok_botton_text), null);
AlertDialog dialog = builder.create();
return dialog;
}
}
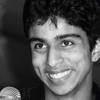
Dhruv Gupta
4,320 PointsPost your code

Akshay Rathee
1,205 Pointspackage bollywoodfacts.myweather; import android.app.AlertDialog; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.util.Log; import com.squareup.okhttp.Callback; import com.squareup.okhttp.OkHttpClient; import com.squareup.okhttp.Request; import com.squareup.okhttp.Call; import com.squareup.okhttp.Response;
import java.io.IOException;
public class MainActivity extends ActionBarActivity { public static final String TAG =MainActivity.class.getSimpleName(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
String apiKey="HIDDEN BY MODERATOR";
double latitude=37.8267;
double longitude= -122.423;
String forecastUrl= "https://api.forecast.io/forecast/"+apiKey+"/"+latitude+","+longitude;
OkHttpClient client= new OkHttpClient();
Request request= new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
Log.v(TAG, response.body().string());
if (response.isSuccessful()) {
}
else {alertUserAboutError();
}
}catch(IOException e){
Log.e(TAG, "Exception Caught;", e);
}
}
}
);
Log.d(TAG, "Main UI code is running");
}
private void alertUserAboutError() {
AlertDialogFragment dialog=new AlertdialogFragment(); dialog.show(getFragmentManager(),"erroe_dialog"); } }
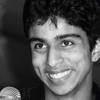
Dhruv Gupta
4,320 PointsOh the answer is pretty clear
You have AlertDialogFragment dialog = new AlertdialogFragment():
Capitalize the lowercase D in the new AlertDialogFragment();
50% of bugs are misspelling or lack of capitalization, They are super annoying, but they are super easy to fix.
Good luck!

Akshay Rathee
1,205 PointsThe problem remains.... Its still showing the same error
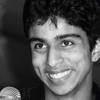
Dhruv Gupta
4,320 PointsYou did create an AlertDialogFragment class right?
You can't import it if it doesn't exist.

Andre Colares
5,437 PointsTry to use my code:
package com.andrecolares.stormy;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import java.io.IOException;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "b58df3c3139b75263e389c950140c157";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude +"," + longitude;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
Log.v(TAG, response.body().string());
if(response.isSuccessful()){
}
else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
Log.d(TAG, "Main UI code is running!");
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
Don't forget to rename your package.

Andre Colares
5,437 Pointspackage com.andrecolares.stormy;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.DialogFragment;
import android.content.Context;
import android.os.Bundle;
public class AlertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context)
.setTitle(R.string.error_title)
.setMessage(R.string.error_message)
.setPositiveButton(R.string.error_ok_button_text, null);
AlertDialog dialog = builder.create();
return dialog;
}
}
Akshay Rathee
1,205 PointsAkshay Rathee
1,205 PointsThanks ....there was a slight error in the class. Now its fixed.