Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial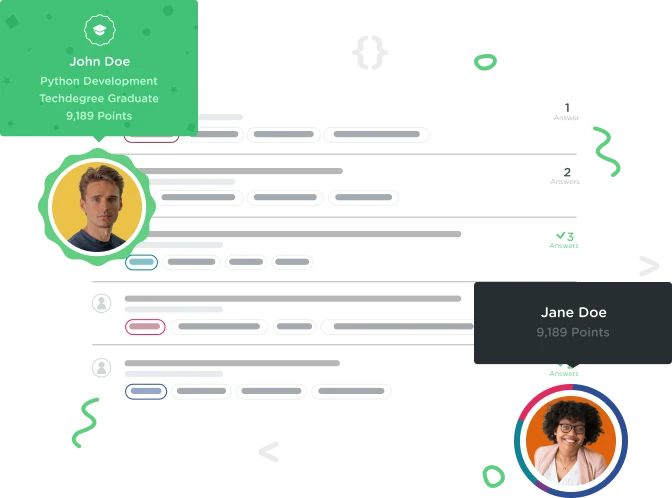

Alexey Papin
iOS Development Techdegree Graduate 26,950 PointsCan't route properly Angular
Trying make REST API for TodoApp written Angular, set routes for ADD, UPDATE, GET ALL, but stuck on DELETE method. My angular controller:
angular.module('todoListApp') .controller('todoCtrl', function($scope, Todo) { $scope.deleteTodo = function(todo, index) { $scope.todos.splice(index, 1); todo.$delete(); console.log("<<" + todo.name + ">> deleted."); };
Angular service:
angular.module('todoListApp') .factory('Todo', function($resource){ return $resource('/todos/', {id: '@id'}, { update: { method: 'PUT' }, save: { method: 'POST' }, delete: { method: 'DELETE', params: {id: '@id'} }
}); });
My Spark controllers (made two for debug) :
delete("/todos", "application/json", (req, res) -> {
Todo todo = gson.fromJson(req.body(), Todo.class);
if (todo == null) throw new ApiError(404, "Could not find todo.");
todoDao.delete(todo);
return todo;
}, gson::toJson);
delete("/todos/:id", "application/json", (req, res) -> {
int id = Integer.parseInt(req.params("id"));
Todo todo = gson.fromJson(req.body(), Todo.class);
if (todo == null) throw new ApiError(404, "Could not find todo.");
todoDao.delete(todo);
return todo;
}, gson::toJson);
When clicking DELETE button (ng-click="deleteTodo(todo, $index)") first controller invoked, but not second. I made two just for debugging. Error message is:
angular.js:10661 DELETE http://localhost:4567/todos?id=3 404 (Not Found)
where I can see right id parameter, but why controller doesnt catch it?
1 Answer

Thomas Nilsen
14,957 PointsIn your backend code (spark), you have the following route:
/todos/:id
Meaning the request should look like this:
http://localhost:4567/todos/3
and not this:
http://localhost:4567/todos?id=3
Your angular should look like something like this:
function deleteTodo(id) {
return $http.delete('http://localhost:4567/todos/' + id)
.then(function(response) {
//some logic here
})
.catch(function(err) {
//Error handling here
});
}
Or alternatively, change your route in spark.