Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial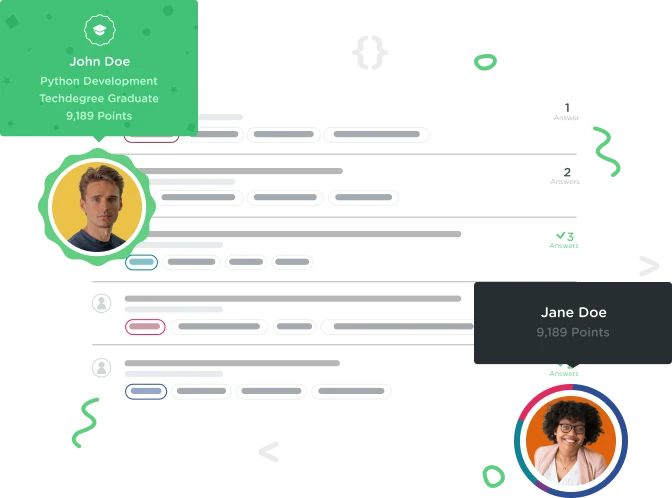
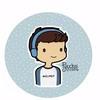
Mihai Mesteru
6,449 PointsCan't run the next page due to an R.java error?
I can't proceed to the next screen - will just show a blank screen. When I run the code, I get this error:
Error:(35, 50) error: cannot find symbol variable choice1Button
This is the code to the StoryActivity.java
package com.misi.interactivestory.ui;
import android.content.Intent;
import android.graphics.drawable.Drawable;
import android.support.v4.content.ContextCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.misi.interactivestory.R;
import com.misi.interactivestory.model.Page;
import com.misi.interactivestory.model.Story;
public class StoryActivity extends AppCompatActivity {
public static final String TAG = StoryActivity.class.getSimpleName();
private Story story;
private ImageView storyImageView;
private TextView storyTextView;
private Button choice1Button;
private Button choice2Button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
storyImageView = (ImageView)findViewById(R.id.storyImageView);
storyTextView = (TextView)findViewById(R.id.storyTextView);
choice1Button = (Button)findViewById(R.id.choice1Button);
choice2Button = (Button)findViewById(R.id.choice2Button);
// get the intent used to start this activity
Intent intent = getIntent();
String name = intent.getStringExtra(getString(R.string.key_name));
if (name == null || name.isEmpty()) {
name = "Friend";
}
Log.d(TAG, name);
story = new Story();
loadPage(0);
}
private void loadPage(int pageNumber) {
Page page = story.getPage(pageNumber);
Drawable image = ContextCompat.getDrawable(this, page.getImageId());
storyImageView.setImageDrawable(image);
}
}
If I could get an y help, that would be great. Thanks!
[MOD: edited code block - srh]
3 Answers
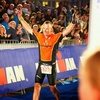
Steve Hunter
57,712 PointsOK - I'm not at my computer but I can see your code. Your xml does not have a button called choice1Button
.
Remember, the Java variable name is separate to the layout name.
You link Java to the layout by assigning a member variable to a xml id.
You need to have a xml id called choice1Button or change your Java to the button id you have already.
Let me know how you get on.
Steve.
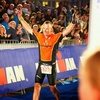
Steve Hunter
57,712 PointsCan you post your XML for the layout, please? If you're getting an R.id issue, it may think that one of your buttons is mis-named in the XML.
Steve.
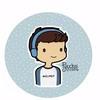
Mihai Mesteru
6,449 PointsFor the Main Activity:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.misi.interactivestory.ui.MainActivity">
<ImageView
android:contentDescription="@string/signals_from_mars_title_image"
android:id="@+id/titleimageView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginLeft="0dp"
android:layout_marginRight="0dp"
android:adjustViewBounds="true"
android:scaleType="fitXY"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:srcCompat="@drawable/main_title"
app:layout_constraintTop_toTopOf="parent"
android:layout_marginTop="0dp"/>
<Button
android:id="@+id/startButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginBottom="0dp"
android:layout_marginLeft="0dp"
android:layout_marginRight="0dp"
android:background="@android:color/white"
android:text="@string/start_your_adventure"
android:textColor="#3A8AEC"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"/>
<EditText
android:id="@+id/nameEditText"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginBottom="0dp"
android:layout_marginLeft="0dp"
android:layout_marginRight="0dp"
android:background="@android:color/white"
android:ems="10"
android:hint="@string/enter_your_name"
android:includeFontPadding="true"
android:inputType="textPersonName"
android:maxLength="30"
app:layout_constraintBottom_toTopOf="@+id/startButton"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"/>
</android.support.constraint.ConstraintLayout>
For the Story Activity:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.misi.interactivestory.ui.StoryActivity">
<Button
android:id="@+id/choice2Button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="#3a8aec"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
tools:text="@string/page0_choice2"/>
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="#3A8AEC"
android:textSize="16sp"
app:layout_constraintBottom_toTopOf="@+id/choice2Button"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
tools:text="@string/page0_choice1"/>
<ScrollView
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:background="@android:color/white"
android:fillViewport="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ImageView
android:contentDescription="@string/story_image_content_description"
android:id="@+id/storyImageView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:adjustViewBounds="true"
android:scaleType="fitXY"
tools:src="@drawable/page0"/>
<TextView
android:id="@+id/storyTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginEnd="16dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:lineSpacingExtra="8sp"
android:textSize="16sp"
tools:text="@string/page0"/>
</LinearLayout>
</ScrollView>
</android.support.constraint.ConstraintLayout>
Mihai Mesteru
6,449 PointsMihai Mesteru
6,449 PointsThat worked - just had to go into the XML and change "@+id/button2" to "choice1button".
Thanks for the help! :)