Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial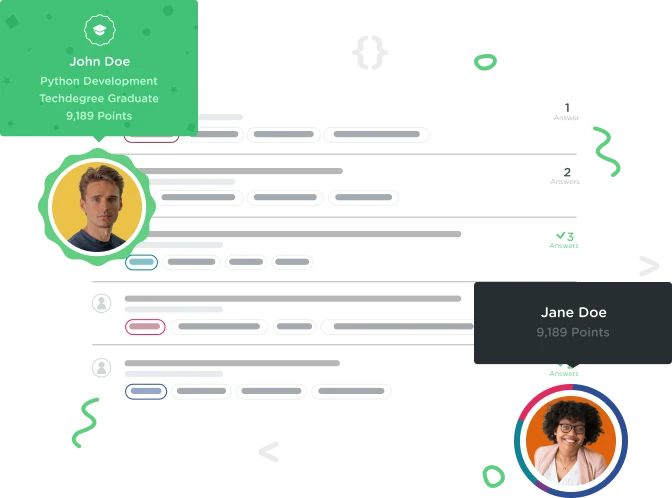
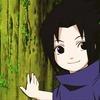
Matthew Francis
6,967 PointsCan't seem to find the purpose of callback functions...
//Without Callback
function mySandwich(param1, param2) {
console.log('Started eating my sandwich.\n\nIt has: ' + param1 + ', ' + param2);
finishSandwich();
}
function finishSandwich(){
console.log('Finished eating my sandwich.');
}
mySandwich("meat", "cheese");
//With Callback
function mySandwich(param1, param2, callback) {
console.log('Started eating my sandwich.\n\nIt has: ' + param1 + ', ' + param2);
callback();
}
function finishSandwich(){
console.log('Finished eating my sandwich.');
}
mySandwich("meat", "cheese", finishSandwich);
I made this code to try to explain the purpose of callbacks. But it seems that, it serves no purpose except for organizational intentions. Am I missing something?
2 Answers
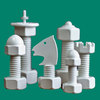
Steven Parker
230,274 PointsThe value of a callback is more obvious in code you don't write.
In this trivial example, you're writing both the callback and the function that uses it. So the value of the callback is not obvious. But if the function that uses the callback were part of a code library that you did not write and do not maintain it would make a big difference.
Here you can easily call finishSandwich directly from within mySandwich because you are the author of both, and you know what you want to happen at the end of finishSandwich. But if mySandwich was part of a library intended for general purpose use, that you neither write nor maintain, the callback would be the only way for you to control what it does at the end.
The value would be equally apparent if you were writing the main function for general use, but you did not know what the final user wanted to happen at the end. But you can accept a callback from them and invoke it when you are finished.
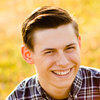
Caleb Kleveter
Treehouse Moderator 37,862 PointsThe example might be better expressed this way:
//Without Callback
function mySandwich(param1, param2) {
console.log('Started eating my sandwich.\n\nIt has: ' + param1 + ', ' + param2);
finishSandwich();
}
function finishSandwich(){
console.log('Finished eating my sandwich.');
}
mySandwich("meat", "cheese");
//With Callback
function mySandwich(param1, param2, callback) {
console.log('Started eating my sandwich.\n\nIt has: ' + param1 + ', ' + param2);
callback();
}
mySandwich("meat", "cheese", function(){
console.log('Finished eating my sandwich.');
});
The callback allows the users of the API to run custom code after the function with the callback is run. This is particularly helpful if you get into asynchronous code.
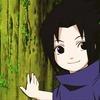
Matthew Francis
6,967 PointsAhh, I just started using API's and this makes absolute sense! What is the syntax for the function in:
mySandwich("meat", "cheese", function(){
console.log('Finished eating my sandwich.');
});
is it called an anonymous function?
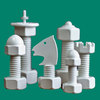
Steven Parker
230,274 PointsThat particular example is an anonymous function, but a callback can also be a named function.