Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial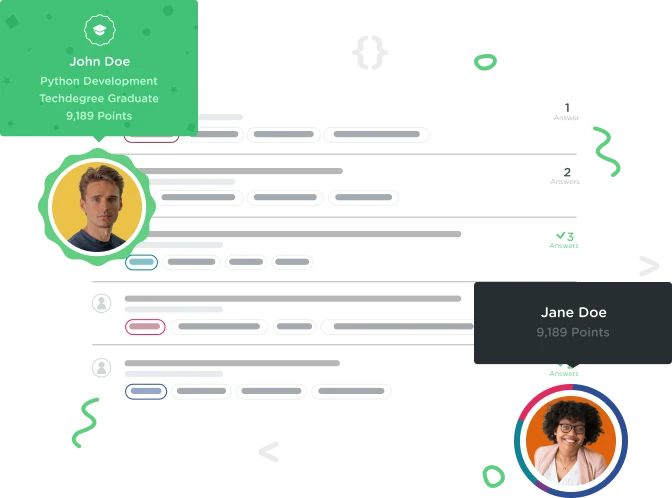

Mike Scott
3,186 PointsCan't set boolean values from default "false" value
Hi all:
I'm a total newbie (only a few days coding), and I'm trying to play around with objects and boolean values. I've created a new instance of my class "cat" with the boolean value set to "false" in my constructor. When I run the "feed" method, I want the boolean value to set to "true". This should be passed as a string in the main class and print out, but instead it remains false. I've noticed that even when I try changing the boolean value to true in the constructor, it remains false, so I'm fairly certain I'm setting the boolean value incorrectly.
Thanks for any help you guys can provide.
Cat class:
class Cat {
private String catName;
public boolean isFed;
public String getIsFed = String.valueOf(isFed);
public Cat(String catName) {
this.catName = catName;
isFed = false;
}
public String getCatName() {
return catName;
}
public void feed() {
isFed = true;
}
public String isHungry() {
return getIsFed;
}
}
Main class -- Example:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
Cat catOne = new Cat("Helen");
System.out.printf("%s is hungry", catOne.getCatName());
catOne.feed();
System.out.printf("Helen is fed: %s", catOne.isHungry());
}
}
1 Answer
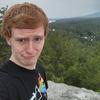
Brendon Butler
4,254 PointsI haven't tested this theory, but it looks like you're setting the "getIsFed" variable only once, you're never changing that value.
for the hunger checker method, I would do this
public boolean isHungry() {
return !isFed; // this will give you the opposite of the variable
}
You should just do this for your second output (also, you can add multiple items to format). I also think you should change "getCatName" to "getName" (just for simplicity sake).
System.out.printf("%s is fed: %b", catOne.getName(), catOne.isHungry());
I would take a look at the conversions section of this document. https://docs.oracle.com/javase/7/docs/api/java/util/Formatter.html#syntax
There are some other things I would probably change, but this is a good start I think.
Mike Scott
3,186 PointsMike Scott
3,186 PointsSo I actually did something a bit different, but your comment got my brain down the right path. I created a new method called "isFull" that checks to see if the "isFed" boolean value == true. I then used that method to print out a response saying my cat is full.
Thanks. Now my cat doesn't have to starve.
Brendon Butler
4,254 PointsBrendon Butler
4,254 PointsSo actually instead of
return isFed == true;
you should just havereturn isFed;
. Since they will give you the same result, but it's no longer redundant.It's kinda like how I changed your "isHungry" getter, but inverted.
EDIT: for only a few days worth of coding, you're doing a great job!