Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial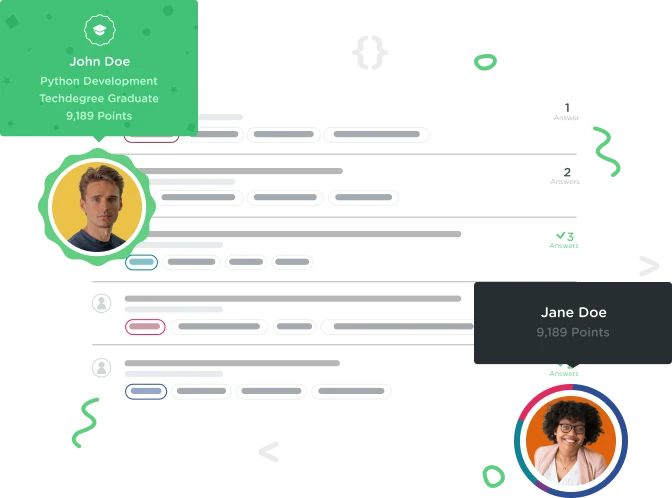

Daniel Kol Adam
3,285 PointsCan't show all student names when the user types in "hack"
My latest attempt here was making a function for displaying all student names and showing them when the user types "hack" yet no matter what it does not show all names, whats the issue with my code?
var message = '';
var student;
var search;
var studentswitch;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
function getStudentName(student) {
for (var i = 0; i < students.length; i += 1) {
var student = students[i];
var message = "<h2> Student: " + student.name + "</h2>";
}
print(message);
}
while (true) {
studentswitch = false;
search = prompt("Search student records: type a name [Jordan] (or type 'quit' to exit)");
if (search === null || search.toLowerCase() === "quit") {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
studentswitch = true;
message = getStudentReport(student);
print(message);
} if (search === "hack") {
getStudentName(student); {
var showAllStudents = "<h2> These are the students: " + student.name + "</h2>";
print(showAllStudents);
} if (!studentswitch) {
var nostudent = "<h2> Student not found! </h2>";
print(nostudent);
}
}
}
}
1 Answer

Greg Kaleka
39,021 PointsHi Daniel,
OK, let's think about this. What do we want the end product to be? When someone enters 'hack', we want to loop through every student name, and gather all of their information, then print it to the screen. To do that, we need to tweak a couple of things:
- How we check to see if a name matches (we want to always return true if 'hack' was entered)
- How we build the message (right now, each time through the for loop, we destroy the message and populate it with a new one)
Here's where Dave left off:
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report
}
while (true) {
search = prompt('Search student records for: (enter a name, or "quit" to quit)');
if (search === null || search.toLocaleLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search) {
message = getStudentReport(student);
print(message);
}
}
}
Step 1: change how we check if the name matches. We want the check to return true if the name matches OR if the name entered was "hack".
// if (student.name === search) {
if (student.name === search || search === 'hack') {
message = getStudentReport(student);
print(message);
}
Step 2: change how we create the message. Right now we're just setting message to the current student information in the loop. Instead, we should build up a message every time we go through a student. To do this we'll keep the message and add to it:
if (student.name === search || search === 'hack') {
// message = getStudentReport(student);
message += getStudentReport(student);
print(message);
}
I hope that makes sense! I know you wanted to just do the names, but I bet you can figure it out from here
Cheers,
-Greg