Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial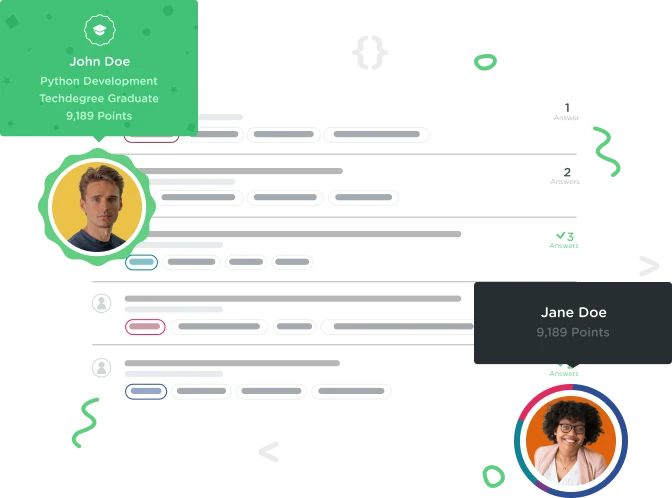

shawn khah
15,082 Pointscan't solve challenge 1 of 1
Challenge Task 1 of 1
SequenceDetector now has a LastScannedSequence property. Change the access of the LastScannedSequence property so that only SequenceDetector and its subclasses can set the LastScannedSequence property. Other classes should still be able to get the last scanned sequence. Important: In each task of this code challenge, the code you write should be added to the code from the previous task. Restart Preview Get Help Check Work SequenceDetector.cs RepeatDetector.cs
1 namespace Treehouse.CodeChallenges 2 { 3 class SequenceDetector 4 { 5 public virtual string Description => ""; 6 β 7 public int[] LastScannedSequence { get; private set; } 8 β 9 public virtual bool Scan(int[] sequence) 10 { 11 LastScannedSequence = sequence; 12 return true; 13 } 14 } 15 } 16 β
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual string Description => "";
public int[] LastScannedSequence { get; private set; }
public virtual bool Scan(int[] sequence)
{
LastScannedSequence = sequence;
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override string Description => "Detects repetitions";
public override bool Scan(int[] sequence)
{
LastScannedSequence = sequence;
if(sequence.Length < 2)
{
return false;
}
for(int i = 1; i < sequence.Length; ++i)
{
if(sequence[i] == sequence[i-1])
{
return true;
}
}
return false;
}
}
}
2 Answers
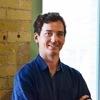
tjgrist
6,553 PointsIt just wants you to change the access level of the property from "private" to "protected" so that the property's set method can still be accessible from it's child classes. So...
Change:
public int[] LastScannedSequence { get; private set; }
To:
public int[] LastScannedSequence { get; protected set; }
Does that make sense why you do that and what the challenge is asking?

shawn khah
15,082 Pointsthank's tj
Alan MattanΓ³
Courses Plus Student 12,188 PointsAlan MattanΓ³
Courses Plus Student 12,188 PointsToo easy answer. If you take out the code, the student will look and search for this "private" and change it to "protected". In any case thanks for the answer!