Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial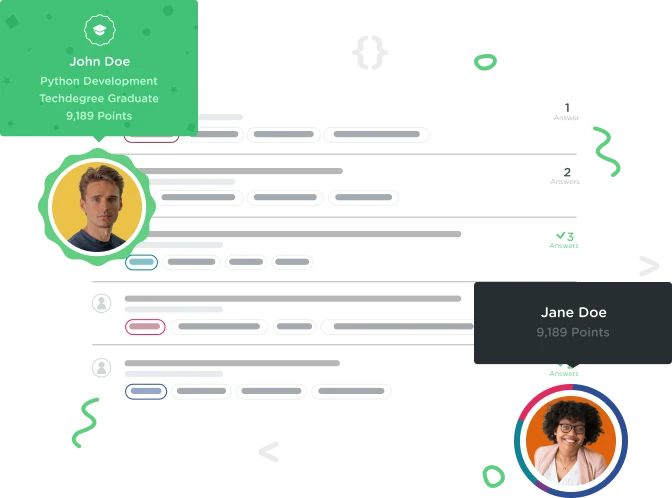

shawn khah
15,082 Pointscan't solve challenge 1 of 1
Challenge Task 1 of 1
Create a static method named GetPowersOf2 that returns a list of powers of 2 from 0 to the value passed in. So if 4 was passed in, the function would return { 1, 2, 4, 8, 16 }. The System.Math.Pow method will come in handy. Bummer! Does MathHelpers.GetPowersOf2 return List`1? Restart Preview Get Help Recheck work MathHelpers.cs
1 using System.Collections.Generic; 2 using System; 3 4 namespace Treehouse.CodeChallenges 5 { 6 public static class MathHelpers 7 { 8 public static List<double> GetPowersOf2(int input) 9 { 10 var returnList = new List<double>(); 11 for (int i = 0; i < input + 1; i++) 12 { 13 returnList.Add(Math.Pow(2, i)); 14 } 15 return returnList; 16 17 } 18 19 } 20 }
using System.Collections.Generic;
using System;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<double> GetPowersOf2(int input)
{
var returnList = new List<double>();
for (int i = 0; i < input + 1; i++)
{
returnList.Add(Math.Pow(2, i));
}
return returnList;
}
}
}
9 Answers
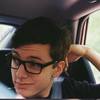
Zachary Steiger
21,391 PointsYou forgot to change your two instances of "List<double>" to "List<int>" on lines 8 and 10, the rest is fine.

Gavin Schilling
37,904 Pointsusing System;
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List <int> GetPowersOf2 (int UpperLimit)
{
Console.WriteLine ("What is the upper limit of the Powers of 2 list you request?");
var PowersOf2List = new List <int> ();
for (int index = 0; index < UpperLimit + 1; index++)
{
PowersOf2List.Add ( (int) Math.Pow (2, index) );
}
return PowersOf2List;
}
}
}
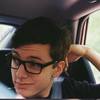
Zachary Steiger
21,391 PointsYou're actually very close, I found your problem when trying to figure out my own code challenge and it made me realize what I had forgotten. When you declare both the list for the GetPowersOf2 method, and the returnList, you must declare int for the list type in order for the challenge to pass. However, Math.Pow only accepts the type double, so you have to use type casting in order to change the Math.Pow return type to int
In short, in addition to changing your two double declarations, all you need to add is the green int below :
returnList.Add((int)Math.Pow(2, i));
Hope this helps, good luck!

shawn khah
15,082 Pointsthank you zack ,but still not working, Challenge Task 1 of 1
Create a static method named GetPowersOf2 that returns a list of powers of 2 from 0 to the value passed in. For example, if 4 is passed in, the method should return this list of integers: { 1, 2, 4, 8, 16 }. The System.Math.Pow method will come in handy. Bummer! Does MathHelpers.GetPowersOf2 return List`1? Restart Preview Get Help Recheck work MathHelpers.cs
1 using System.Collections.Generic; 2 using System; 3 4 namespace Treehouse.CodeChallenges 5 { 6 public static class MathHelpers 7 { 8 public static List<double> GetPowersOf2(int input) 9 { 10 var returnList = new List<double>(); 11 for (int i = 0; i < input + 1; i++) 12 { 13 returnList.Add((int)Math.Pow(2, i)); 14 } 15 return returnList; 16 17 } 18 19 } 20 }
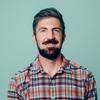
Ford Heacock
18,068 PointsThis was very helpful for me as well, thanks Zachary!
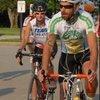
Gerald Tinney
15,454 Pointsvery close, try changing List<double> to List<int> then change returnList to....
returnList.Add((int)System.Math.Pow(2, i));

Carel Du Plessis
Courses Plus Student 16,356 PointsMy answer to the Challenge
using System;
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<int> GetPowersOf2(int num)
{
int listSizes = num + 1;
double list;
List<int> math = new List<int>(listSizes);
for (int i = 0; i < math.Capacity; i++)
{
list = Math.Pow(2, i);
math.Add(Convert.ToInt32(list));
}
return math;
}
}
}

Kevin Gates
15,053 PointsMy answer:
using System.Collections.Generic;
using System;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<int> GetPowersOf2 (int value)
{
List<int> returnList = new List<int>(value+1);
for (int i = 0 ; i < returnList.Capacity; i++)
{
returnList.Add(Convert.ToInt32(Math.Pow(2, i)));
}
return returnList;
}
}
}

Damacious White
4,647 Pointspublic static List<int> GetPowersOf2(int listLenghth) { List<int> powers = new List<int>(listLenghth + 1); for (int i = 0; i < powers.Capacity; i++) { powers.Add((int)Math.Pow(2, i)); }
return powers;
}
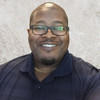
Donte Taylor
4,457 PointsHere's the code that worked for me:
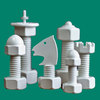
Steven Parker
230,688 Points
The challenge is apparently expecting a list of integers to be returned.
They probably should have mentioned that. You might want to report it as a bug on the Support page.
shawn khah
15,082 Pointsshawn khah
15,082 Pointsawesome,thank you so much zack