Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial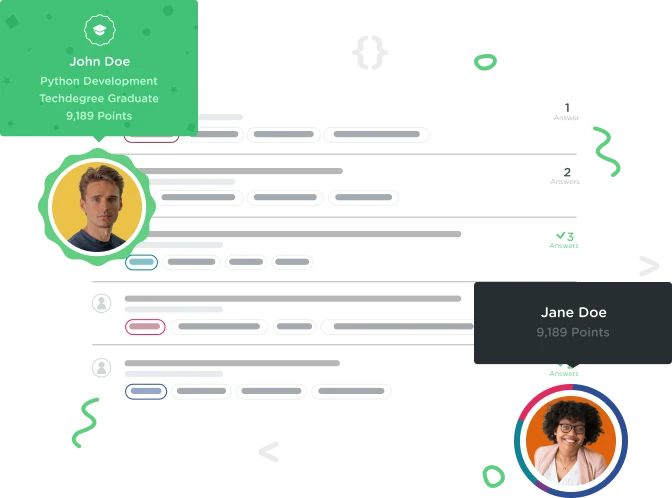

Jamie Baldaro
15,616 PointsCan't solve Challenge 2 of 2
I thought I understood all of the getter/setter bits, but apparently something i've done isn't quite right?
Does anyone have any idea what i've done wrong? Thanks
namespace Treehouse.CodeChallenges
{
class Frog
{
private int _numFliesEaten;
public int GetNumFliesEaten()
{
return _numFliesEaten;
}
public void SetNumFliesEaten( _numFliesEaten value)
{
_numFliesEaten = value;
}
}
}
3 Answers

Lukas Dahlberg
53,736 PointsIn the arguments for your setter, you have _numFliesEaten value. You need to set the type and the argument name. (And you should set the argument name as something different than the private field.)
So, it should be:
public void SetNumFliesEaten( int numFliesEaten)
{
_numFliesEaten = numFliesEaten;
}

Daniel W
1,341 PointsBe careful! The special word "value" is used when you have a set method for a property, not regular methods:
class Frog
{
private int _numFliesEaten;
public int NumFliesEaten
{ //A property with get and set methods, replacing the ones you wrote.
private get //We can tag the getter or setter with the private keyword to limit access from other classes.
{
return _numFliesEaten;
}
set
{
_numFliesEaten = value;
Scream(value); //Here value is whatever was passed when we change NumFliesEaten.
}
}
private void Scream(int amount)
{
Console.WriteLine("I have eaten " + amount + " flies!");
}
}
Somewhere else in another class we could have:
Frog frog = new Frog();
frog.NumFliesEaten = 3; //This would call the set of the property of the Frog class, the "value" variable will be 3.
frog._numFliesEaten = 3; //Wouldn't work, since the variable is private.
var somevalue = frog.NumFliesEaten //Wouldn't work, we have a private getter. This other class can't read the value.
a short and simple Property would be:
private int _numFliesEaten; //This can be removed.
public int NumFliesEaten{get;set;} //And we don't even have to bother with returns etc. In this case both get and set are public.
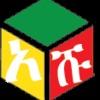
Ashenafi Ashebo
15,021 Pointsuse "int" type in the setter method, not _numFliesEaten.
namespace Treehouse.CodeChallenges { class Frog { private int _numFliesEaten; public int GetNumFliesEaten () { return _numFliesEaten; }
public void SetNumFliesEaten(int value)
{
_numFliesEaten = value;
}
}
} use "int" type in the setter method, not _numFliesEaten.
Jamie Baldaro
15,616 PointsJamie Baldaro
15,616 PointsThat's brilliant. thank you :)
Feel like that was an obvious mistake and i just failed to see it >.<